C#: Reverse a specified array of integers and length 5
Reverse Array
Write a C# Sharp program to reverse a given array of integers and length 5.
Visual Presentation:
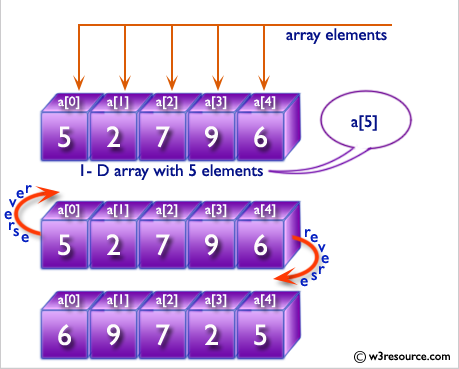
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with an integer array as an argument and assigning the result to the 'item' array
int[] item = test(new[] { 10, 20, -30, -40, 50 });
// Outputting text to indicate the start of displaying the reverse array
Console.Write("Reverse array: ");
// Looping through each element of the 'item' array and displaying them
foreach(var i in item)
{
Console.Write(i.ToString()+" "); // Displaying each element of the 'item' array separated by a space
}
}
// Method to reverse the order of elements in an integer array
public static int[] test(int[] nums)
{
// Returning a new array with elements in reverse order
return new int[] { nums[4], nums[3], nums[2], nums[1], nums[0] };
}
}
}
Sample Output:
Reverse array: 50 -40 -30 20 10
Flowchart:
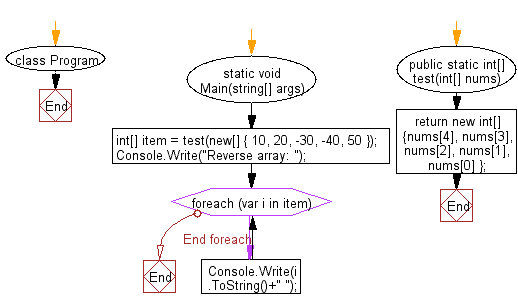
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to rotate the elements of a given array of integers (length 4 ) in left direction and return the new array.
Next: Write a C# Sharp program to find out the maximum element between the first or last element in a given array of integers( length 4), replace all elements with maximum elelment.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics