C#: Check a given array of integers of length 1 or more and return true if the first element and the last element are equal in the given array
First and Last Array Elements Equal
Write a C# Sharp program to check a given array of integers of length 1 or more. Return true if the first and the last element in the array are equal.
Visual Presentation:
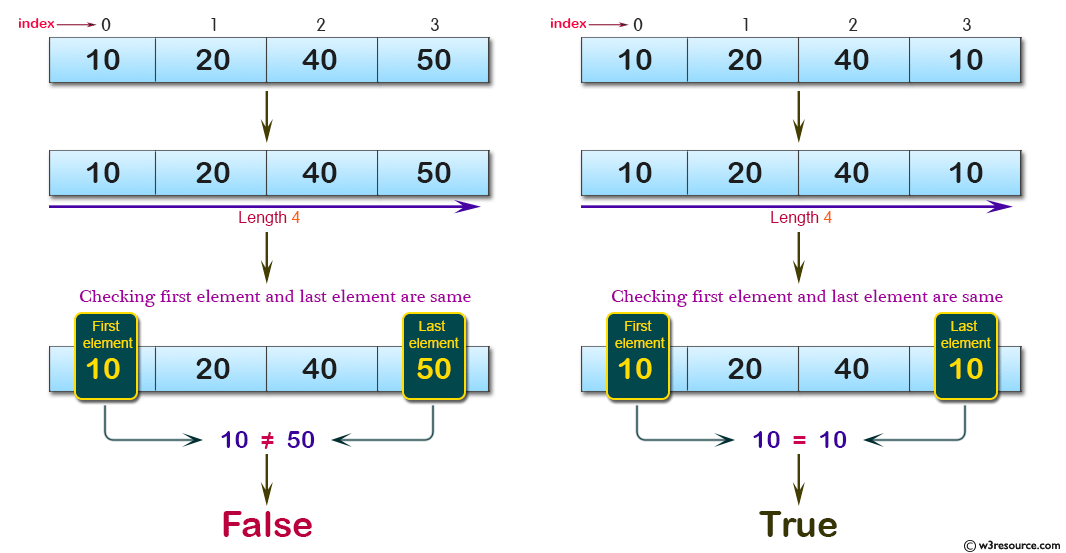
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the results of the 'test' function with different integer arrays as arguments
Console.WriteLine(test(new[] { 10, 20, 40, 50 })); // Calling test method with array [10, 20, 40, 50] and displaying the result
Console.WriteLine(test(new[] { 10, 20, 40, 10 })); // Calling test method with array [10, 20, 40, 10] and displaying the result
Console.WriteLine(test(new[] { 12, 24, 35, 55 })); // Calling test method with array [12, 24, 35, 55] and displaying the result
Console.ReadLine(); // Waiting for user input before closing the console window
}
// Method to check if the first and last elements of an array are equal
public static bool test(int[] nums)
{
// Returns true if the array length is greater than 0 and the first and last elements are equal
return nums.Length > 0 && nums[0] == nums[nums.Length - 1];
}
}
}
Sample Output:
False True False
Flowchart:
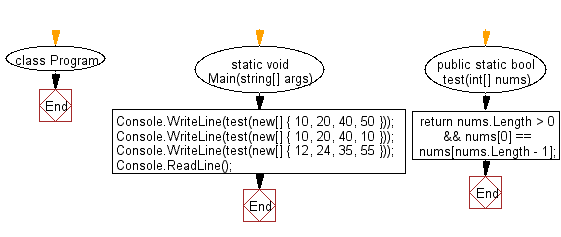
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check a given array of integers of length 1 or more and return true if 10 appears as either first or last element in the given array
Next: Write a C# Sharp program to check two given arrays of integers of length 1 or more and return true if they have the same first element or they have the same last element.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.