C#: Create a new string of length 2, using first two characters of a given string
First Two Characters with Missing '#' if Short
Write a C# Sharp program to create a new string of length 2, using the first two characters of a given string. If the given string length is less than 2 use '#' as missing characters.
Visual Presentation:
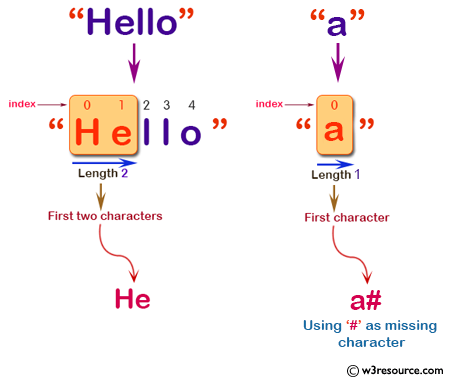
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the results of the test function with different strings as arguments
Console.WriteLine(test("Hello")); // Pass "Hello" as an argument to the test function
Console.WriteLine(test("Python")); // Pass "Python" as an argument to the test function
Console.WriteLine(test("a")); // Pass "a" as an argument to the test function
Console.WriteLine(test("")); // Pass an empty string as an argument to the test function
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of the test function that takes a string 's1' and returns a string
public static string test(string s1)
{
if (s1.Length >= 2) // Check if the length of 's1' is greater than or equal to 2
{
s1 = s1.Substring(0, 2); // If so, take the substring of 's1' starting from index 0 with a length of 2
}
else if (s1.Length > 0) // Check if 's1' length is greater than 0 but less than 2
{
s1 = s1.Substring(0, 1) + "#"; // If so, take the first character of 's1' and concatenate "#" to it
}
else // If 's1' is an empty string
{
s1 = "##"; // Assign 's1' as "##"
}
return s1; // Return the modified or original 's1'
}
}
}
Sample Output:
He Py a# ##
Flowchart:
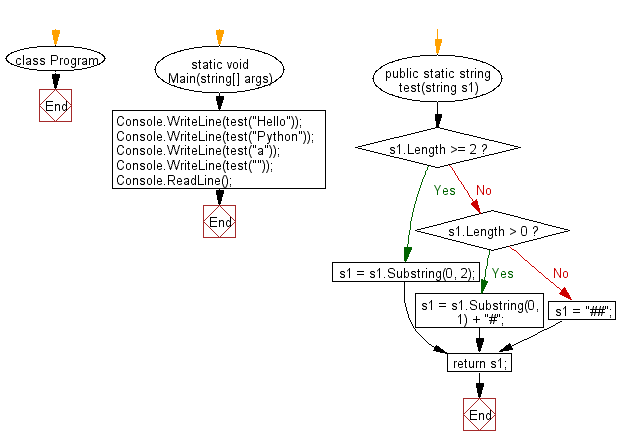
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new string taking 3 characters from the middle of a given string at least 3.
Next: Write a C# Sharp program to create a new string taking the first character from a given string and the last character from another given string. If the length of any given string is 0, use '#' as its missing character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics