C#: Insert a given string into middle of the another given string of length 4
Insert String into Middle of Another
Write a C# Sharp program to insert a given string into the middle of another given string of length 4.
Visual Presentation:
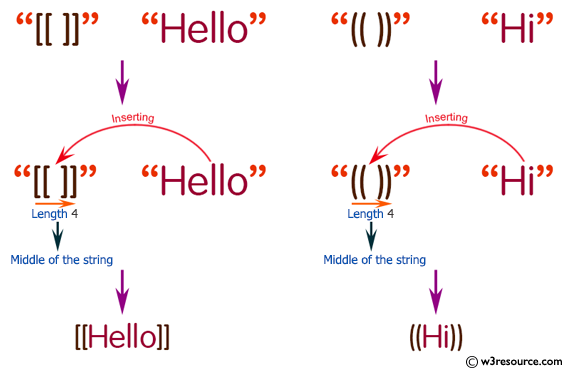
Sample Solution:-
C# Sharp Code:
using System; // Import the System namespace which contains fundamental types and base classes
using System.Collections.Generic; // Import the System.Collections.Generic namespace for collections
using System.Linq; // Import the LINQ namespace for LINQ functionality
using System.Text; // Import the System.Text namespace for string manipulation
using System.Threading.Tasks; // Import the System.Threading.Tasks namespace for asynchronous operations
namespace exercises // Define a namespace called 'exercises'
{
class Program // Define a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of test function with two strings as arguments
Console.WriteLine(test("[[]]", "Hello")); // Test with strings "[[]]" and "Hello"
Console.WriteLine(test("(())", "Hi")); // Test with strings "(())" and "Hi"
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of test function that takes two strings 's1' and 'word' and returns a string
public static string test(string s1, string word)
{
// Concatenates a substring of s1 (from index 0 to index 2 exclusive) with the 'word' and then concatenates the rest of s1 (from index 2 onwards)
return s1.Substring(0, 2) + word + s1.Substring(2);
}
}
}
Sample Output:
[[Hello]] ((Hi))
Flowchart:
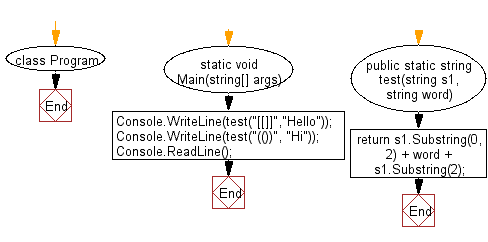
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new string using two given strings s1, s2, the format of the new string will be s1s2s2s1.
Next: create a new string using three copies of the last two character of a given string of length at least two.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.