C#: Create a new string where 'if' is added to the front of a given string
Add 'if' to String if Absent
Write a C# Sharp program to create a string where 'if' is added to the front of a given string. If the string already begins with 'if', return it unchanged.
Visual Presentation:
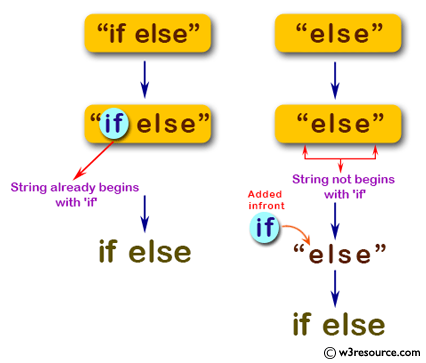
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different strings and displaying the returned values
Console.WriteLine(test("if else")); // Output: "if else"
Console.WriteLine(test("else")); // Output: "if else"
Console.ReadLine(); // Keeping the console window open
}
// Method to modify the string based on a condition
public static string test(string s)
{
// Checking if the length of the string is greater than 2 and if the substring from index 0 to 1 is "if"
if (s.Length > 2 && s.Substring(0, 2).Equals("if"))
{
return s; // If the condition is true, return the original string 's'
}
return "if " + s; // If the condition is false, prepend "if " to the string 's' and return it
}
}
}
Sample Output:
if else if else
Flowchart:
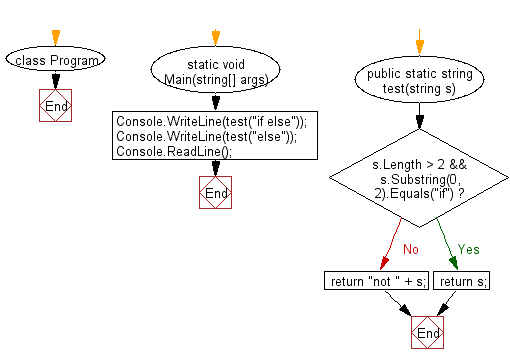
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check a given integer and return true if it is within 10 of 100 or 200.
Next: Write a C# Sharp program to remove the character in a given position of a given string. The given position will be in the range 0.str.length()-1 inclusive).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics