C#: Compute the sum of the two given integers
Sum in Range 10-20 Returns 30
Write a C# Sharp program to compute the sum of the two given integers. If the sum is in the range 10..20 inclusive return 30.
Visual Presentation:
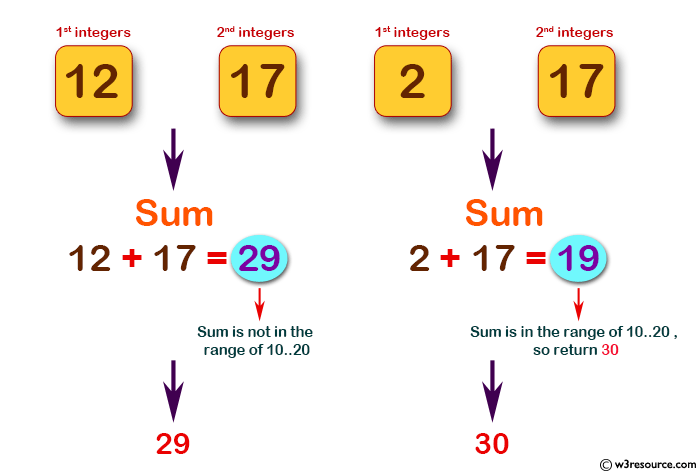
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different integers and printing the results
Console.WriteLine(test(12, 17)); // Output: 30
Console.WriteLine(test(2, 17)); // Output: 19
Console.WriteLine(test(22, 17)); // Output: 30
Console.WriteLine(test(20, 0)); // Output: 30
Console.ReadLine(); // Keeping the console window open
}
// Method to check the sum of two integers and return a specific value based on conditions
public static int test(int a, int b)
{
// Check if the sum of 'a' and 'b' is between 10 and 20 (inclusive)
// If true, return 30; otherwise, return the actual sum of 'a' and 'b'
return a + b >= 10 && a + b <= 20 ? 30 : a + b;
}
}
}
Sample Output:
29 30 39 30
Flowchart:
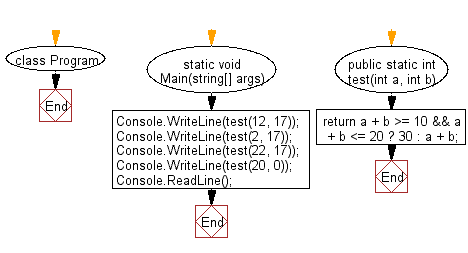
For more Practice: Solve these Related Problems:
- Write a C# program that returns 50 if the sum of two numbers falls within the range 30 to 60 inclusive.
- Write a C# program to return the absolute sum of two numbers unless the sum is between 15 and 25, then return zero.
- Write a C# program to return a fixed value (like 100) if either number is in the 10–20 range and the sum is not.
- Write a C# program to return the sum of two integers, but if both are in range 10–20, return double their sum.
Go to:
PREV : Check Triple in Array.
NEXT : Check for 5 or Sum/Difference Equals 5.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.