C#: Create a new string of the characters at indexes 0,1, 4,5, 8,9 ... from a given string
Characters at Index 0,1,4,5,...
Write a C# Sharp program to create a string of characters at indexes 0,1, 4,5, 8,9 ... from a given string.
Visual Presentation:
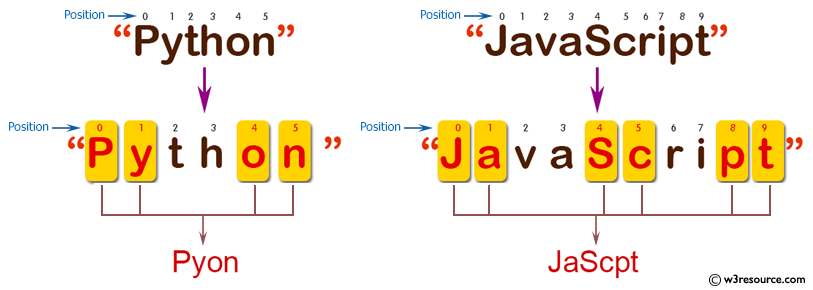
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different strings
Console.WriteLine(test("Python")); // Output: Py
Console.WriteLine(test("JavaScript")); // Output: Ja
Console.WriteLine(test("HTML")); // Output: HT
Console.ReadLine(); // Keeping the console window open
}
// Method to process a string and extract substrings at specific intervals
public static string test(string str1)
{
var result = string.Empty; // Initializing an empty string to store the result
for (var i = 0; i < str1.Length; i += 4) // Loop through the characters of the string with a step of 4
{
var c = i + 2; // Calculate the index position to start the substring
var n = 0; // Initialize a variable to hold the length of the substring
n += c > str1.Length ? 1 : 2; // Determine the length based on the position and length of the string
result += str1.Substring(i, n); // Extract a substring and append it to the result
}
return result; // Return the final result after processing the string
}
}
}
Sample Output:
Pyon JaScpt HT
Flowchart:
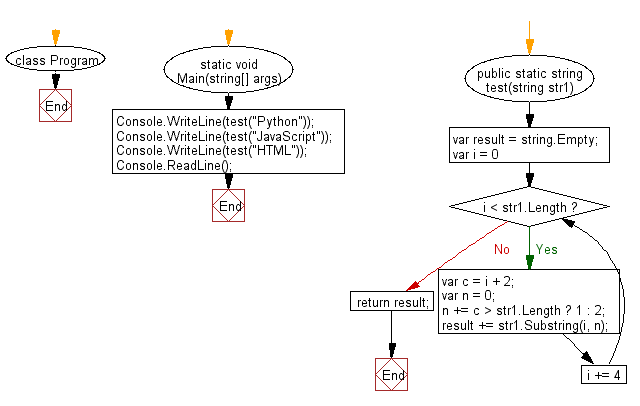
For more Practice: Solve these Related Problems:
- Write a C# program to build a string using characters at positions 0,2,4,6,8,... from a given string.
- Write a C# program to create a new string using every 4th character group of 2 characters (e.g., 0,1 then 4,5 etc.).
- Write a C# program to generate a string from indexes 0,3,6,... and 1,4,7,... interleaved together.
- Write a C# program to extract characters from every third block of 3 characters starting from the beginning of a string.
Go to:
PREV : Remove Character Except First and Last.
NEXT : Count 5's Next to Each Other.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.