C#: Count a substring of length 2 appears any where in a given string as well as appears at the end of the string
Count Substring Matching Last Two Characters
Write a C# Sharp program to count a substring of length 2 that appears in a given string. This substring appears as the last 2 characters of the string. Do not count the end substring.
Visual Presentation:
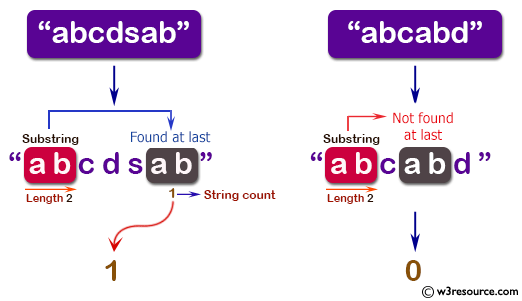
Sample Solution:-
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different string inputs
Console.WriteLine(test("abcdsab")); // Output: 2
Console.WriteLine(test("abcdabab")); // Output: 3
Console.WriteLine(test("abcabdabab")); // Output: 4
Console.WriteLine(test("abcabd")); // Output: 0
Console.ReadLine(); // Keeping the console window open
}
// Method to count occurrences of the last two characters in substrings
public static int test(string str)
{
var last_two_char = str.Substring(str.Length - 2); // Getting the last two characters of the input string
var ctr = 0; // Counter to store the number of occurrences
// Loop through the string except for the last two characters
for (var i = 0; i < str.Length - 2; i++)
{
// Check if the substring of length 2 starting from index 'i' matches the last two characters
if (str.Substring(i, 2).Equals(last_two_char))
{
ctr++; // Increment the counter if the condition is met
}
}
return ctr; // Return the count of occurrences
}
}
}
Sample Output:
1 2 3 0
Flowchart:
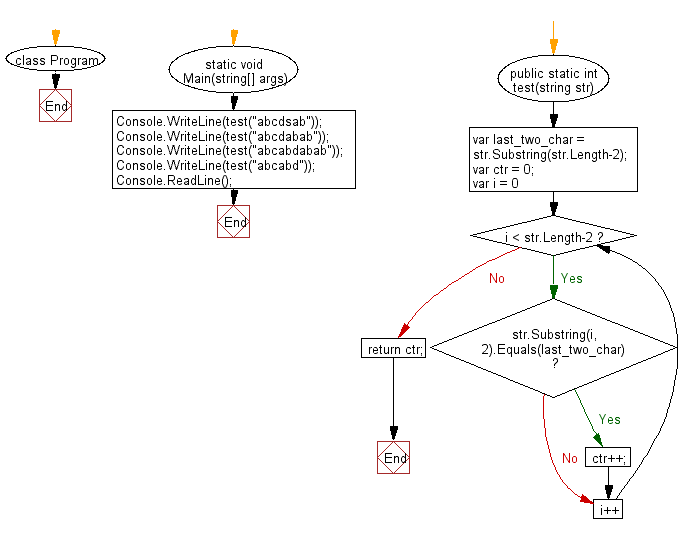
For more Practice: Solve these Related Problems:
- Write a C# program to count how many times a substring of length 2 appears in the first half of the string and matches the last 2 characters.
- Write a C# program to count all substrings of length 2 that match any repeated pattern in the second half of the string, excluding the last.
- Write a C# program to count matching 2-character substrings with the last two characters, skipping overlapping matches.
- Write a C# program to count matches for every substring of length 2 that also appears at both the start and end of the string.
Go to:
PREV : Write a C# Sharp program to create a string like "aababcabcd" from a given string "abcd".
NEXT : Check if Element Present in Array.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.