C#: Check two given integers, and return true if one of them is 30 or if their sum is 30
Check 30 or Sum Equals 30
Write a C# Sharp program to check two given integers, and return true if one of them is 30 or if their sum is 30.
Visual Presentation:
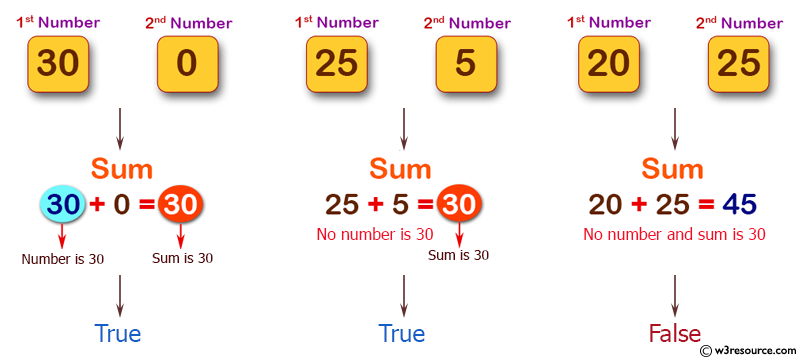
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method and displaying the returned boolean values
Console.WriteLine(test(30, 0)); // Output: True
Console.WriteLine(test(25, 5)); // Output: True
Console.WriteLine(test(20, 30)); // Output: True
Console.WriteLine(test(20, 25)); // Output: False
Console.ReadLine(); // Keeping the console window open
}
// Method to check conditions based on two integer parameters x and y
public static bool test(int x, int y)
{
// Returning true if any of the conditions are met:
// 1. x equals 30
// 2. y equals 30
// 3. The sum of x and y equals 30
return x == 30 || y == 30 || (x + y == 30);
}
}
}
Sample Output:
True True True False
Flowchart:
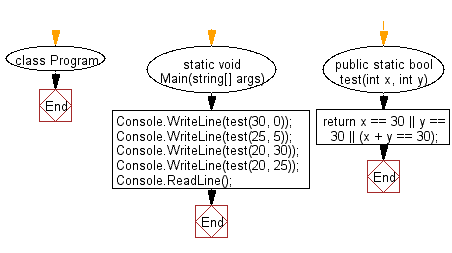
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to get the absolute difference between n and 51. If n is greater than 51 return triple the absolute difference.
Next: Write a C# Sharp program to check a given integer and return true if it is within 10 of 100 or 200.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.