C#: Create a new string which is n copies of a given string
n Copies of String
Write a C# Sharp program to create a string which is n (non-negative integer) copies of a given string.
Visual Presentation:
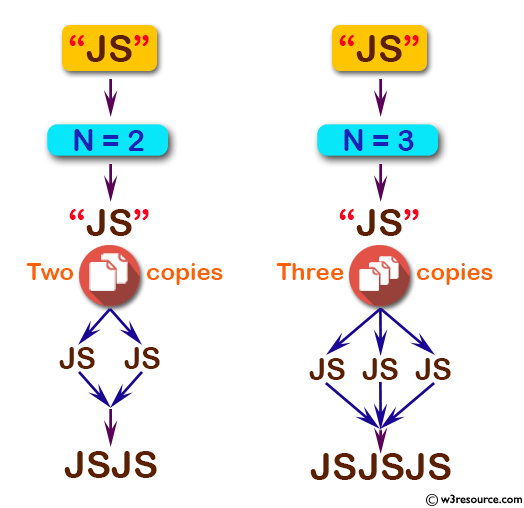
Sample Solution:-
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different string inputs and integers
Console.WriteLine(test("JS", 2)); // Output: JSJS
Console.WriteLine(test("JS", 3)); // Output: JSJSJS
Console.WriteLine(test("JS", 1)); // Output: JS
Console.ReadLine(); // Keeping the console window open
}
// Method to concatenate a string 's' 'n' times
public static string test(string s, int n)
{
string result = String.Empty; // Initialize an empty string to store the result
// Loop 'n' times to concatenate the input string 's'
for (int i = 0; i < n; i++)
{
result += s; // Concatenate the input string to the result string 'n' times
}
return result; // Return the concatenated string
}
}
}
Sample Output:
JSJS JSJSJS JS
Flowchart:
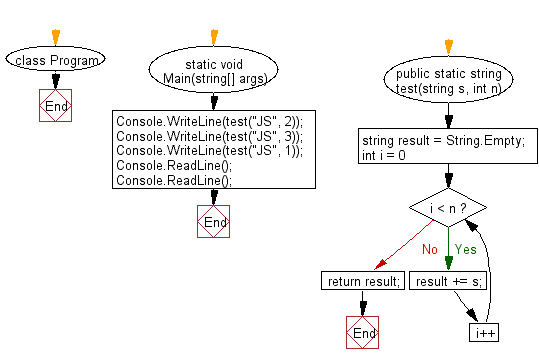
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to convert the last 3 characters of a given string in upper case. If the length of the string has less than 3 then uppercase all the characters.
Next: Write a C# Sharp program to create a new string which is n (non-negative integer )copies of the the first 3 characters of a given string.If the length of the given string is less than 3 then return n copies of the string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.