C#: Check whether two given integers are in the range 40..50 inclusive, or they are both in the range 50..60 inclusive
Both Integers in Ranges 40-50 or 50-60
Write a C# Sharp program to check whether two given integers are in the range 40..50 inclusive, or they are both in the range 50..60 inclusive.
Visual Presentation:
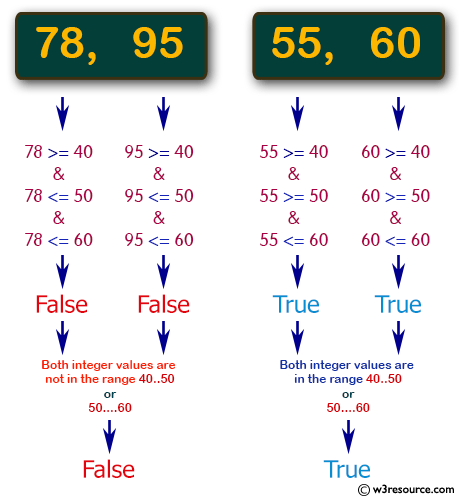
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different integer values
Console.WriteLine(test(78, 95)); // Output: True
Console.WriteLine(test(25, 35)); // Output: False
Console.WriteLine(test(40, 50)); // Output: True
Console.WriteLine(test(55, 60)); // Output: True
Console.ReadLine(); // Keeping the console window open
}
// Method to check if the given coordinates (x, y) fall within specified ranges
public static bool test(int x, int y)
{
// Checking if both x and y fall within the first range (x: [40, 50], y: [40, 50])
// or if both x and y fall within the second range (x: [50, 60], y: [50, 60])
return (x >= 40 && x <= 50 && y >= 40 && y <= 50) || (x >= 50 && x <= 60 && y >= 50 && y <= 60);
}
}
}
Sample Output:
False False True True
Flowchart:
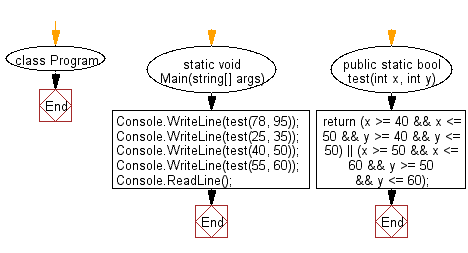
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: check which number nearest to the value 100 among two given integers. Return 0 if the two numbers are equal.
Next: Write a C# Sharp program to find the larger value from two positive integer values that is in the range 20..30 inclusive, or return 0 if neither is in that range.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.