C#: Create a new list from a given list of integers removing those values end with 7
Remove Integers Ending in 7
Write a C# Sharp program to create a list from a given list of integers, removing all values ending in 7.
Visual Presentation:
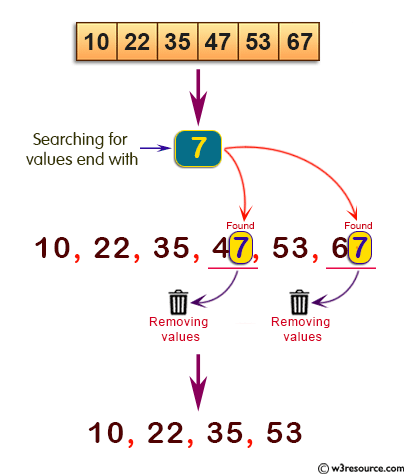
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace for basic functionality
using System.Collections.Generic; // Importing the namespace for working with collections
using System.Linq; // Importing the namespace for LINQ operations
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with a new list of integers as input
List<int> mylist = test(new List<int>(new int[] { 10, 22, 35, 47, 53, 67 }));
// Displaying elements of 'mylist' in the console
foreach(var i in mylist)
{
Console.Write(i.ToString()+" "); // Output each element followed by a space
}
}
// Method that filters a List of integers to include only those whose last digit is less than 7
public static List<int> test(List<int> nums)
{
// Using LINQ to filter 'nums' list and select elements whose last digit is less than 7
return nums.Where(n => n % 10 < 7).ToList();
}
}
}
Sample Output:
10 22 35 53
Flowchart:
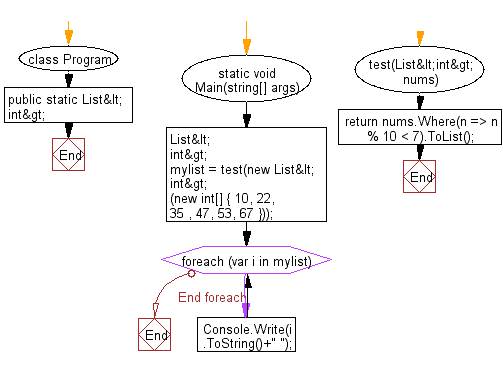
Go to:
PREV : Remove Integers Less Than 4.
NEXT : C# Exception Handling Exercises Home.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.