C#: Create a new list from a given list of integers where each integer multiplied by itself three times
C# Sharp Basic Algorithm: Exercise-142 with Solution
Write a C# Sharp program to create a list from a given list of integers. Each integer is multiplied by itself three times.
Visual Presentation:
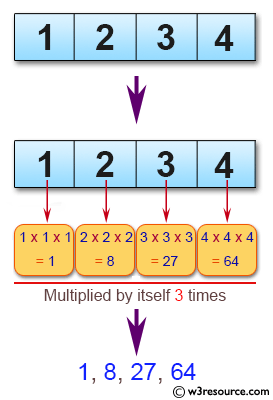
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace for basic functionality
using System.Collections.Generic; // Importing the namespace for working with collections
using System.Linq; // Importing the namespace for LINQ operations
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Creating a List of integers and assigning the result of 'test' method
List<int> mylist = test(new List<int>(new int[] { 1, 2, 3 , 4 }));
// Displaying the elements of 'mylist' in the console
foreach (var i in mylist)
{
Console.Write(i.ToString() + " "); // Output each element followed by a space
}
}
// Method that takes a List of integers and returns a new List with each element cubed
public static List<int> test(List<int> nums)
{
// Using LINQ to cube each element of 'nums' and store it in 'squared'
IEnumerable<int> squared = nums.Select(x => x * x * x);
// Converting the IEnumerable 'squared' to a new List and returning it
return squared.ToList();
}
}
}
Sample Output:
1 8 27 64
Flowchart:
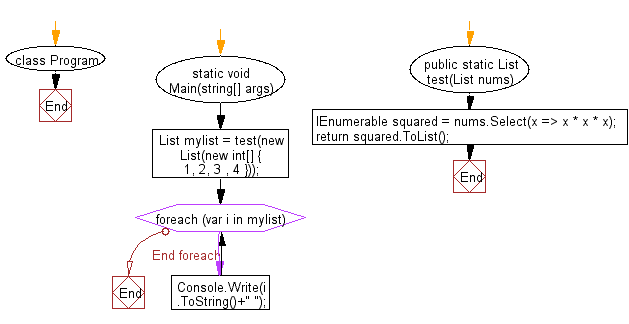
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new list from a given list of integers where each element is multiplied by 3.
Next: Write a C# Sharp program to create a new list from a given list of strings where each element has "#" added at the beginning and end position.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-142.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics