C#: Count the number of strings of specified length in a given array of strings
Count Strings Matching Length
Write a C# Sharp program to count the number of strings with given lengths in a given array of strings.
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace for basic functionality
using System.Collections; // Importing collections for non-generic interfaces
using System.Collections.Generic; // Importing collections for generic interfaces
using System.Linq; // Importing LINQ for querying collections
using System.Text; // Importing classes for handling strings
using System.Threading.Tasks; // Importing classes for handling asynchronous tasks
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Prompting the user for input
Console.WriteLine("Number of Strings:");
// Calling the 'test' method with an array of strings and a specified length
Console.WriteLine(test(new[] {"a", "b", "bb", "c", "ccc" }, 1));
}
// Method to count the number of strings with a specific length in the array
static int test(string[] arr_str, int len)
{
int ctr = 0; // Counter to track the number of strings with the specified length
// Looping through each string in the array
for (int i = 0; i < arr_str.Length; i++)
{
// Checking if the length of the current string matches the specified length
if (arr_str[i].Length == len)
{
ctr++; // Incrementing the counter if the length matches
}
}
return ctr; // Returning the total count of strings with the specified length
}
}
}
Sample Output:
Number of Strings: 3
Flowchart:
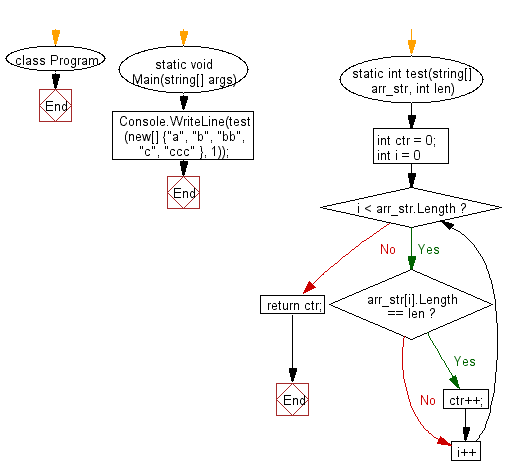
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to find the larger average value between the first and the second half of a given array of integers and minimum length is atleast 2. Assume that the second half begins at index (array length)/2.
Next: Write a C# Sharp program to create a new array using the first n strings from a given array of strings. (n>=1 and <=length of the array)
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics