C#: Return true if there are two values 15, 15 next to each other in an array
Check for Two 15's Next to Each Other
Write a C# Sharp program to check a given array (length will be at least 2) of integers and return true if there are two values 15, 15 next to each other.
Visual Presentation:
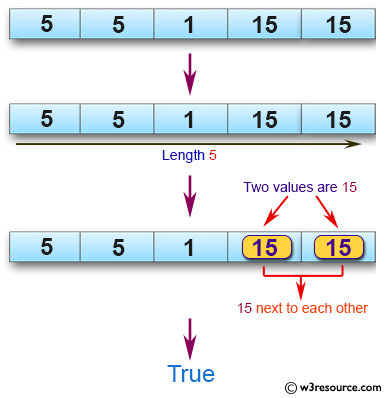
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the result of the 'test' method with various integer arrays
Console.WriteLine(test(new[] { 5, 5, 1, 15, 15 }));
Console.WriteLine(test(new[] { 15, 2, 3, 4, 15 }));
Console.WriteLine(test(new[] { 3, 3, 15, 15, 5, 5 }));
Console.WriteLine(test(new[] { 1, 5, 15, 7, 8, 15 }));
}
// Method to check if an array has consecutive occurrences of number 15
static bool test(int[] numbers)
{
for (int i = 0; i < numbers.Length - 1; i++) // Loop through the array
{
if (numbers[i + 1] == numbers[i] && numbers[i] == 15) // Checking if two consecutive elements are both 15
{
return true; // If found, return true
}
}
return false; // If the loop completes without finding two consecutive 15s, return false
}
}
}
Sample Output:
True False True False
Flowchart:
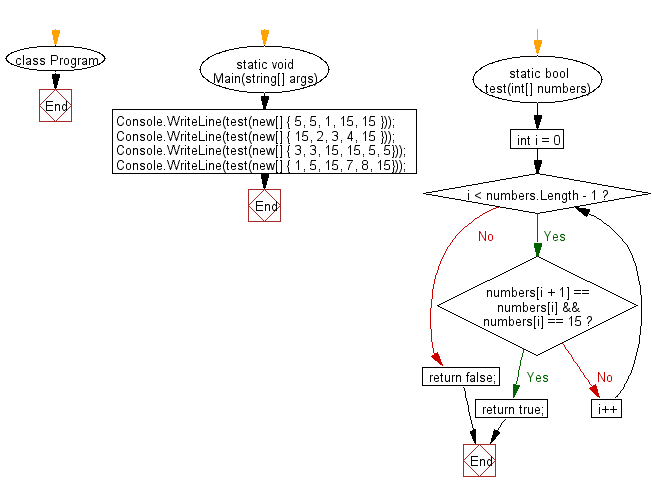
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check if the value of each element is equal or greater than the value of previous element of a given array of integers.
Next: Write a C# Sharp program to find the larger average value between the first and the second half of a given array of integers and minimum length is atleast 2. assume that the second half begins at index (array length)/2.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics