C#: Create a new array taking the elements before the element value 5 from a given array of integers
Elements Before 5 in Array
Write a C# Sharp program to create an array taking the elements before 5 from a given array of integers.
Visual Presentation:
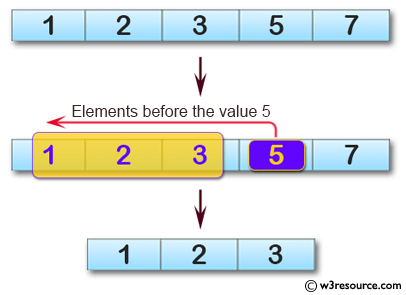
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with a given integer array and storing the returned array in 'item'
int[] item = test(new[] { 1, 2, 3, 5, 7 });
// Outputting the elements of the new array formed before the element '5' if found
Console.Write("New array: ");
foreach (var i in item) // Looping through each element in the 'item' array
{
Console.Write(i.ToString() + " "); // Outputting each element followed by a space
}
}
// Method to extract elements of an array before the occurrence of the value '5'
static int[] test(int[] numbers)
{
int size = 0; // Variable to store the size of the elements before '5'
int[] pre_ele_5; // Array to hold the elements before '5'
// Looping through the array to find the index where '5' occurs
for (int i = 0; i < numbers.Length; i++)
{
if (numbers[i] == 5)
{
size = i; // Storing the index where '5' is found
break;
}
}
pre_ele_5 = new int[size]; // Creating an array with size determined before '5'
// Storing elements before '5' into the pre_ele_5 array
for (int j = 0; j < size; j++)
{
pre_ele_5[j] = numbers[j];
}
return pre_ele_5; // Returning the array containing elements before '5'
}
}
}
Sample Output:
New array: 1 2 3
Flowchart:
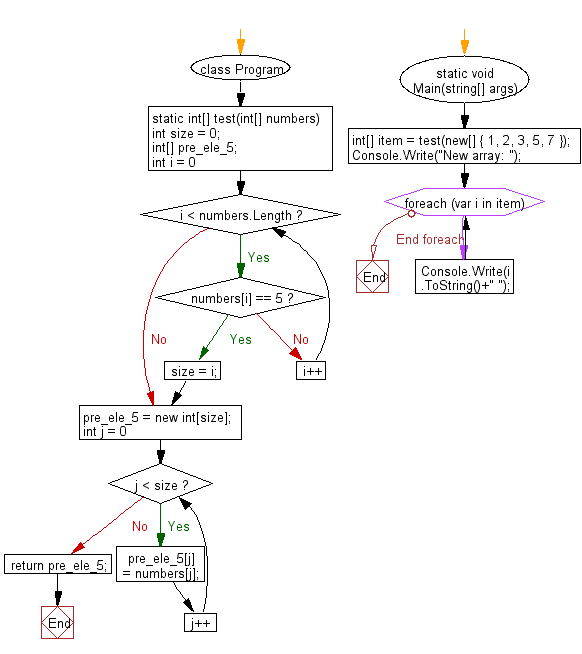
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to shift an element in left direction and return a new array.
Next: Write a C# Sharp program to create a new array taking the elements after the element value 5 from a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.