C#: Check a given array of integers and return true if the array contains three increasing adjacent numbers
C# Sharp Basic Algorithm: Exercise-126 with Solution
Write a C# Sharp program to check a given array of integers and return true if the array contains three increasing adjacent numbers.
Visual Presentation:
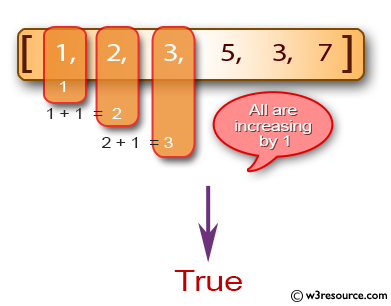
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the results of the 'test' method with different integer arrays as arguments
Console.WriteLine(test(new[] { 1, 2, 3, 5, 3, 7 })); // Testing the method with an array
Console.WriteLine(test(new[] { 3, 7, 5, 5, 3, 7 })); // Testing the method with an array
Console.WriteLine(test(new[] { 3, 7, 5, 5, 6, 7, 5 })); // Testing the method with an array
}
// Method to check if there are three consecutive elements in the array
static bool test(int[] numbers)
{
for (int i = 0; i <= numbers.Length - 3; i++) // Loop through the array elements until the third-to-last element
{
// Checking if three consecutive elements form a sequence (incrementing by 1)
if (numbers[i] == numbers[i + 1] - 1 && numbers[i] == numbers[i + 2] - 2)
{
return true; // Return true if a sequence of three consecutive elements is found
}
}
return false; // Return false if no sequence of three consecutive elements is found
}
}
}
Sample Output:
True False True
Flowchart:
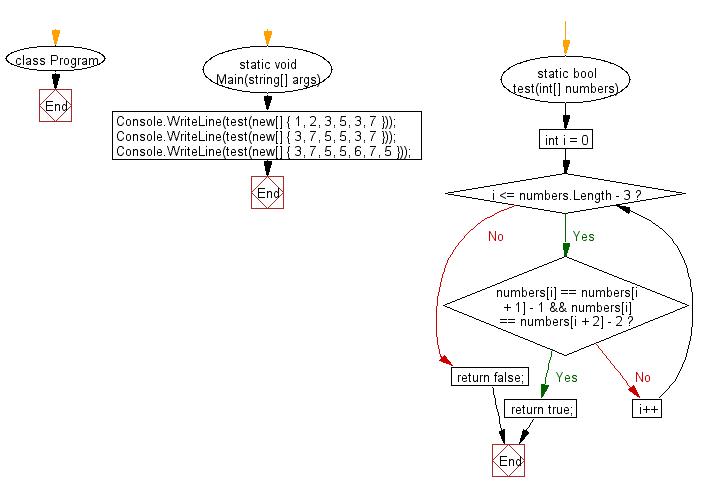
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check a given array of integers and return true if the specified number of same elements appears at the start and end of the given array.
Next: Write a C# Sharp program to shift an element in left direction and return a new array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics