C#: Create a new array from two given array of integers, each length 3
Merge Two Arrays of Length 3
Write a C# Sharp program to create an array from two arrays of integers, each length 3.
Visual Presentation:
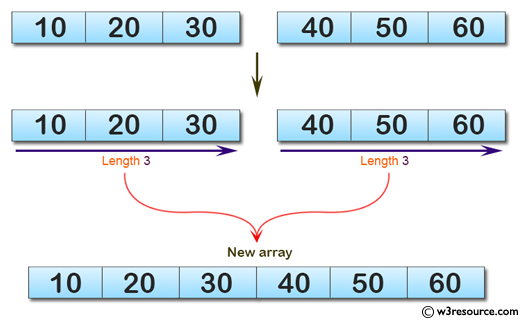
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with two integer arrays as arguments and assigning the result to the 'item' array
int[] item = test(new[] { 10, 20, 30 }, new[] { 40, 50, 60 });
// Outputting text to indicate the start of displaying the new array
Console.Write("New array: ");
// Looping through each element of the 'item' array and displaying them
foreach(var i in item)
{
Console.Write(i.ToString()+" "); // Displaying each element of the 'item' array separated by a space
}
}
// Method to combine two arrays into a new array containing elements from both arrays
public static int[] test(int[] nums1, int[] nums2)
{
// Creating a new array with the elements of 'nums1' followed by the elements of 'nums2'
return new int[] { nums1[0], nums1[1], nums1[2], nums2[0], nums2[1], nums2[2] };
}
}
}
Sample Output:
New array: 10 20 30 40 50 60
Flowchart:
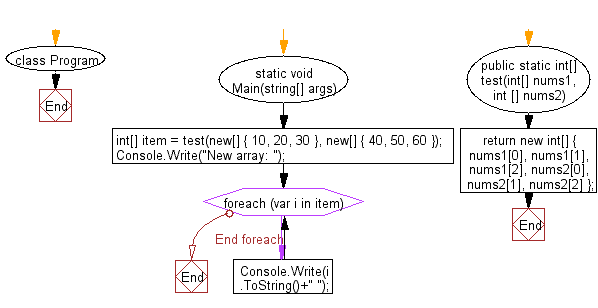
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create an array taking two middle elements from a given array of integers of length even.
Next: Write a C# Sharp program to create a new array swapping the first and last elements of a given array of integers and length will be least 1.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.