C#: Compute the sum of the two given integer values
Triple Sum for Equal Integers
Write a C# Sharp program to compute the sum of the two numerical values. If the two values are the same, return triple their sum.
Visual Presentation:
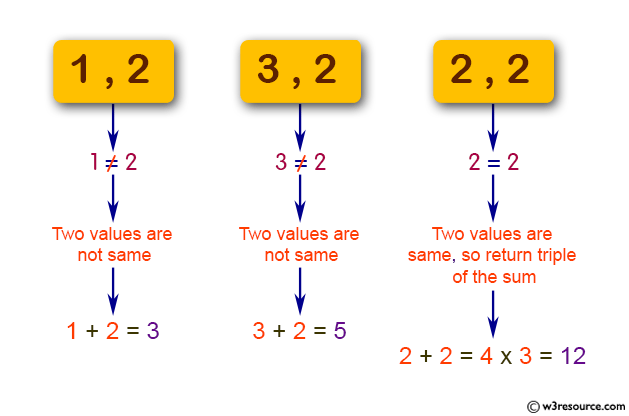
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method and displaying the returned values
Console.WriteLine(test(1, 2)); // Output: 3
Console.WriteLine(test(3, 2)); // Output: 5
Console.WriteLine(test(2, 2)); // Output: 12
Console.ReadLine(); // Keeping the console window open
}
// Method to perform a calculation based on the conditions
public static int test(int x, int y)
{
// Conditional operator to check if x is equal to y
// If true, return the sum of x and y multiplied by 3, else return the sum of x and y
return x == y ? (x + y) * 3 : x + y;
}
}
}
Sample Output:
3 5 12
Flowchart:
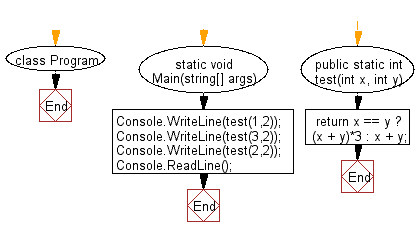
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: C# Sharp Basic Declarations and Expressions Home
Next: Write a C# Sharp program to get the absolute difference between n and 51. If n is greater than 51 return triple the absolute difference.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.