C#: Count frequency of each element of an array
Write a C# Sharp program to count the frequency of each element in an array.
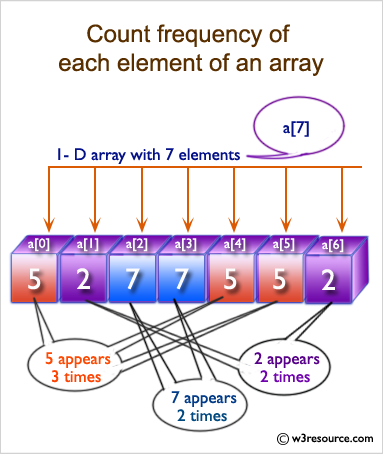
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise8
{
public static void Main()
{
int[] arr1 = new int[100]; // Array to store elements
int[] fr1 = new int[100]; // Array to store frequency
int n, i, j, ctr; // Variables for size, loop control, and frequency counting
Console.Write("\n\nCount the frequency of each element of an array:\n");
Console.Write("----------------------------------------------------\n");
Console.Write("Input the number of elements to be stored in the array :");
n = Convert.ToInt32(Console.ReadLine()); // Read the number of elements
Console.Write("Input {0} elements in the array :\n", n);
for (i = 0; i < n; i++)
{
Console.Write("element - {0} : ", i);
arr1[i] = Convert.ToInt32(Console.ReadLine()); // Read elements into the array
fr1[i] = -1; // Initialize frequency array with -1
}
// Count frequency of each element
for (i = 0; i < n; i++)
{
ctr = 1; // Initialize counter to 1
for (j = i + 1; j < n; j++)
{
if (arr1[i] == arr1[j]) // If current element matches other elements
{
ctr++; // Increment frequency counter
fr1[j] = 0; // Mark duplicates by setting their frequency to 0
}
}
if (fr1[i] != 0) // If not a duplicate
{
fr1[i] = ctr; // Store the frequency
}
}
// Display frequency of each element
Console.Write("\nThe frequency of all elements of the array : \n");
for (i = 0; i < n; i++)
{
if (fr1[i] != 0) // If frequency is not 0 (indicating duplicate)
{
Console.Write("{0} occurs {1} times\n", arr1[i], fr1[i]); // Display element and its frequency
}
}
}
}
Sample Output:
Count the frequency of each element of an array: ---------------------------------------------------- Input the number of elements to be stored in the array :3 Input 3 elements in the array : element - 0 : 10 element - 1 : 15 element - 2 : 10 The frequency of all elements of the array : 10 occurs 2 times 15 occurs 1 times
Flowchart:
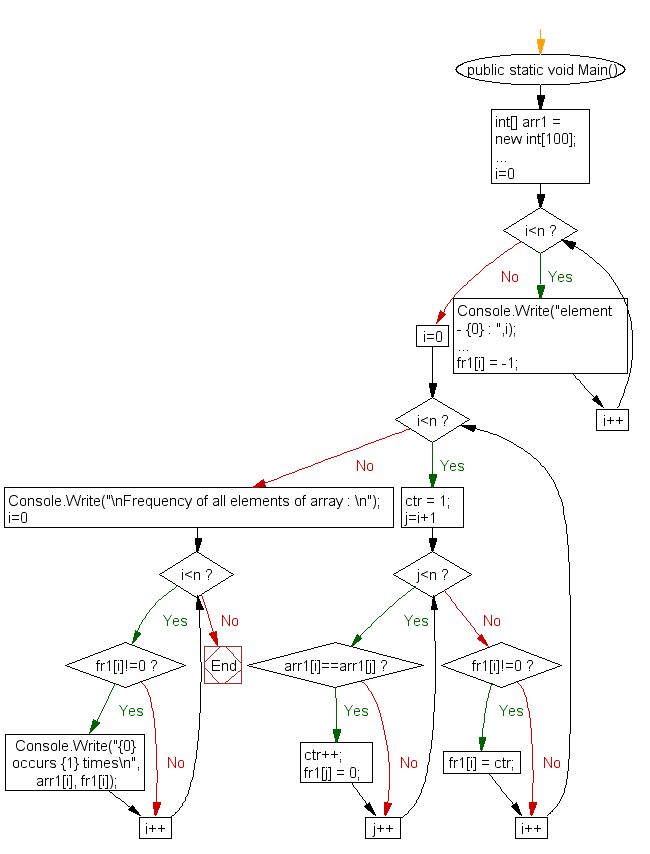
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to merge two arrays of same size sorted in ascending order.
Next: Write a program in C# Sharp to find maximum and minimum element in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.