C#: Merge two arrays of same size sorted in ascending order
Write a C# Sharp program to merge two arrays of the same size sorted in ascending order.
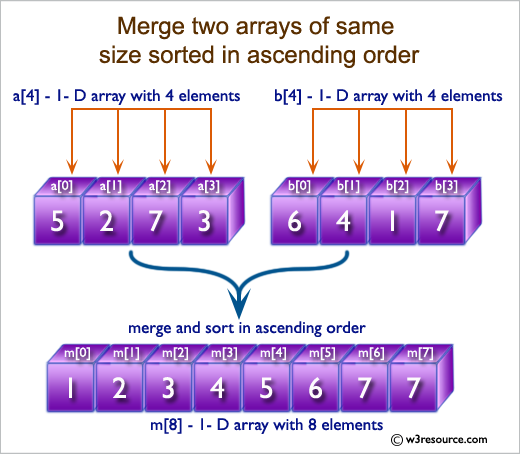
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise7
{
public static void Main()
{
int[] arr1 = new int[100]; // First array
int[] arr2 = new int[100]; // Second array
int[] arr3 = new int[200]; // Merged array
int s1, s2, s3; // Sizes of arrays
int i, j, k; // Loop control variables
Console.Write("\n\nMerge two arrays of same size sorted in ascending order.\n");
Console.Write("------------------------------------------------------------\n");
Console.Write("Input the number of elements to be stored in the first array :");
s1 = Convert.ToInt32(Console.ReadLine()); // Read size of the first array
Console.Write("Input {0} elements in the array :\n", s1); // Prompt to input elements for the first array
for (i = 0; i < s1; i++)
{
Console.Write("element - {0} : ", i);
arr1[i] = Convert.ToInt32(Console.ReadLine()); // Read elements for the first array
}
Console.Write("Input the number of elements to be stored in the second array :");
s2 = Convert.ToInt32(Console.ReadLine()); // Read size of the second array
Console.Write("Input {0} elements in the array :\n", s2); // Prompt to input elements for the second array
for (i = 0; i < s2; i++)
{
Console.Write("element - {0} : ", i);
arr2[i] = Convert.ToInt32(Console.ReadLine()); // Read elements for the second array
}
// Calculate size of the merged array
s3 = s1 + s2;
// Merge the two arrays into the third array
for (i = 0; i < s1; i++)
{
arr3[i] = arr1[i]; // Copy elements from the first array to the merged array
}
for (j = 0; j < s2; j++)
{
arr3[i] = arr2[j]; // Append elements from the second array to the merged array
i++;
}
// Sort the merged array in ascending order using bubble sort algorithm
for (i = 0; i < s3; i++)
{
for (k = 0; k < s3 - 1; k++)
{
if (arr3[k] >= arr3[k + 1])
{
j = arr3[k + 1];
arr3[k + 1] = arr3[k];
arr3[k] = j;
}
}
}
// Print the merged array in ascending order
Console.Write("\nThe merged array in ascending order is :\n");
for (i = 0; i < s3; i++)
{
Console.Write("{0} ", arr3[i]);
}
Console.Write("\n\n");
}
}
Sample Output:
Merge two arrays of same size sorted in ascending order. ------------------------------------------------------------ Input the number of elements to be stored in the first array :2 Input 2 elements in the array : element - 0 : 1 element - 1 : 2 Inpu2 the number of elements to be stored in the second array :2 Input 2 elements in the array : element - 0 : 3 element - 1 : 4 The merged array in ascending order is : 1 2 3 4
Flowchart:
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to print all unique elements in an array.
Next: Write a program in C# Sharp to count the frequency of each element of an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics