C#: Count a total number of duplicate elements in an array
C# Sharp Array: Exercise-5 with Solution
Write a C# Sharp program in to count duplicate elements in an array.
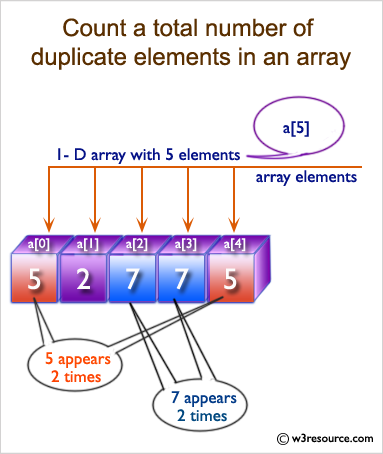
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise5 // Declaration of the Exercise5 class
{
public static void Main() // Main method, entry point of the program
{
int[] arr1 = new int[100]; // Declaration of an integer array 'arr1' with size 100
int[] arr2 = new int[100]; // Declaration of another integer array 'arr2' with size 100
int[] arr3 = new int[100]; // Declaration of another integer array 'arr3' with size 100
int s1, mm = 1, ctr = 0; // Declaration of variables 's1', 'mm', and 'ctr'
int i, j; // Declaration of loop control variables 'i' and 'j'
// Display a message to count the total number of duplicate elements in an array
Console.Write("\n\nCount total number of duplicate elements in an array:\n");
Console.Write("---------------------------------------------------------\n");
Console.Write("Input the number of elements to be stored in the array :");
s1 = Convert.ToInt32(Console.ReadLine()); // Read the number of elements from the user and store it in 's1'
Console.Write("Input {0} elements in the array:\n", s1); // Prompt the user to input 's1' elements
for (i = 0; i < s1; i++)
{
Console.Write("element - {0} : ", i);
arr1[i] = Convert.ToInt32(Console.ReadLine()); // Read user input and store it in the array 'arr1'
}
// Copy elements from 'arr1' to 'arr2' and initialize 'arr3' with 0
for (i = 0; i < s1; i++)
{
arr2[i] = arr1[i]; // Copy each element from 'arr1' to 'arr2'
arr3[i] = 0; // Initialize 'arr3' with 0
}
// Mark the elements that are duplicate in 'arr3'
for (i = 0; i < s1; i++)
{
for (j = 0; j < s1; j++)
{
if (arr1[i] == arr2[j])
{
arr3[j] = mm; // Mark duplicate elements in 'arr3' with 'mm'
mm++; // Increment 'mm'
}
}
mm = 1; // Reset 'mm' to 1
}
// Count the number of duplicate elements in 'arr3'
for (i = 0; i < s1; i++)
{
if (arr3[i] == 2)
{
ctr++; // Increment 'ctr' for each duplicate element found in 'arr3'
}
}
Console.Write("The number of duplicate elements is: {0} \n", ctr); // Output the count of duplicate elements
Console.Write("\n\n"); // Extra newline for formatting
}
}
Sample Output:
Count total number of duplicate elements in an array: --------------------------------------------------------- Input the number of elements to be stored in the array :3 Input 3 elements in the array : element - 0 : 2 element - 1 : 2 element - 2 : 4 Total number of duplicate elements found in the array is : 1
Flowchart:
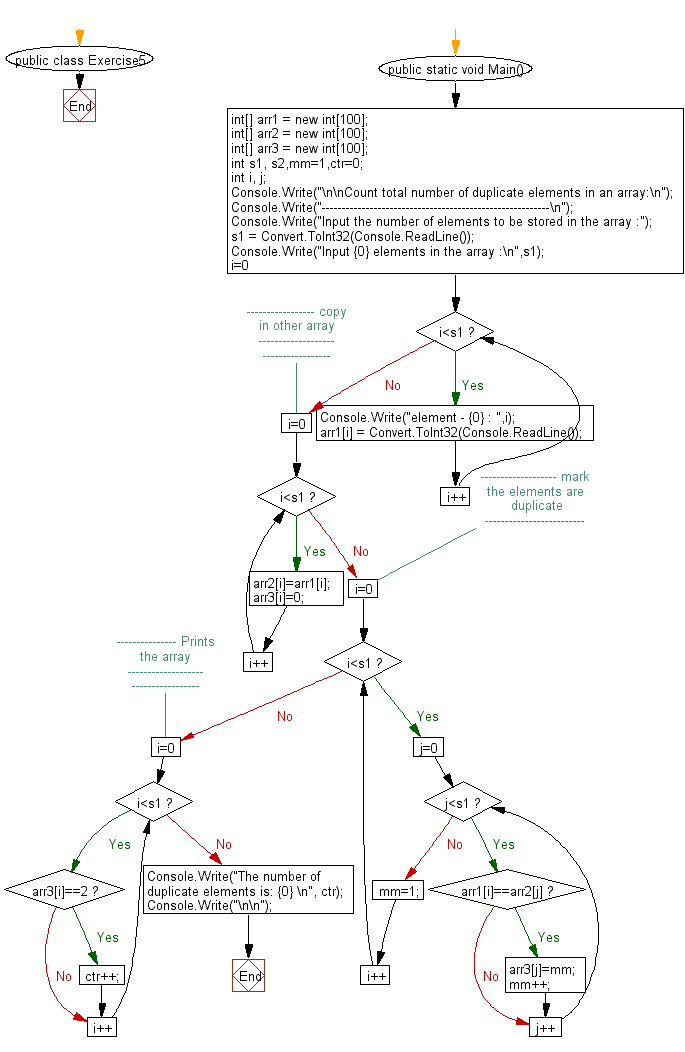
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to copy the elements one array into another array.
Next: Write a program in C# Sharp to print all unique elements in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/array/csharp-array-exercise-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics