C#: Smallest positive which is not present in an array
C# Sharp Array: Exercise-40 with Solution
Write a C# Sharp program that takes an array of integers and finds the smallest positive integer that is not present in that array.
Sample Data:
({ 1,2,3,5,6,7}) -> 4
({-1, -2, 0, 1, 3, 4, 5, 6}) -> 2
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing an array of integers
int[] nums1 = { 1, 2, 3, 5, 6, 7 };
// Displaying original array elements using string.Join method
Console.WriteLine("Original array elements:");
Console.WriteLine($"{string.Join(", ", nums1)}");
// Finding and displaying the smallest positive number not present in the array
Console.WriteLine("Smallest positive number which is not present in an array: " + test(nums1));
// Creating another array of integers
int[] nums2 = { -1, -2, 0, 1, 3, 4, 5, 6 };
Console.WriteLine("\nOriginal array elements:");
Console.WriteLine($"{string.Join(", ", nums2)}");
// Finding and displaying the smallest positive number not present in the second array
Console.WriteLine("Smallest positive number which is not present in an array: " + test(nums2));
}
// Method to find the smallest positive number not present in the array
public static int test(int[] nums)
{
int i = 1;
// Loop until the smallest positive number not present in the array is found
while (true)
{
// Check if the current value of 'i' is not present in the array
if (!(nums.Count(v => v == i) > 0))
{
// If 'i' is not present in the array, return it as the smallest positive number
return i;
}
// Increment 'i' to check the next positive number
i++;
}
}
}
}
Sample Output:
Original array elements: 1, 2, 3, 5, 6, 7 Smallest positive which is not present in an array: 4 Original array elements: -1, -2, 0, 1, 3, 4, 5, 6 Smallest positive which is not present in an array: 2
Flowchart:
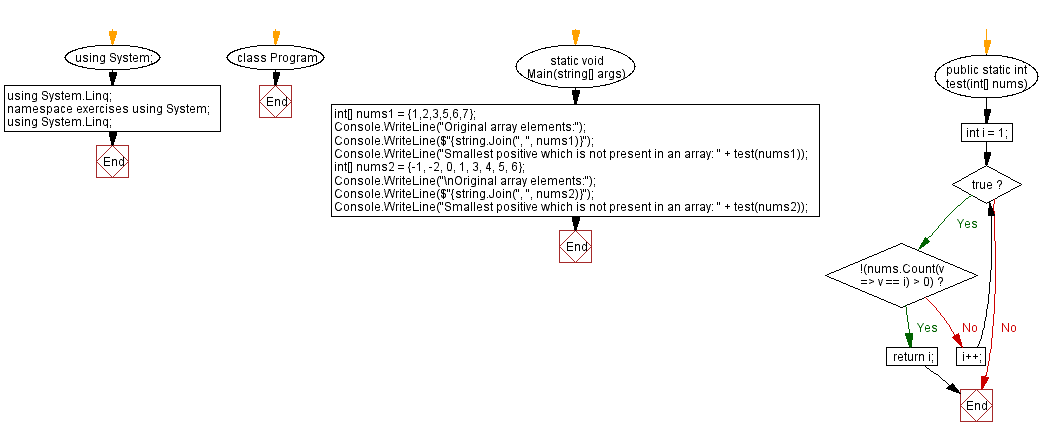
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous C# Sharp Exercise: Sum of all prime numbers in an array.
Next C# Sharp Exercise: Find the product of two integers in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/array/csharp-array-exercise-40.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics