C#: Check specific digit in an array of numbers
Write a C# Sharp program that takes an array of numbers and a digit. Check whether the digit is present in this array of numbers.
Sample Data:
({7, 5, 85, 9, 11, 23, 18}, "1") - > "Present"
({7, 5, 85, 9, 11, 23, 18}, "1") - > "Present"
({7, 5, 85, 9, 11, 23, 18}, "1") - > "Not present..!"
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing an array of integers
int[] nums = { 7, 5, 85, 9, 11, 23, 18 };
// Initializing a string representing a digit to search for
string n = "1";
// Displaying original array elements using string.Join method
Console.WriteLine("Original array elements:");
Console.WriteLine($"{string.Join(", ", nums)}");
// Displaying the digit to search for
Console.WriteLine("\nDigit to search: " + n);
// Checking if the specified digit is present in the array and displaying the result
Console.WriteLine("Check if the said digit is present in the array: " + test(nums, n));
// Changing the digit to search for and repeating the process
n = "9";
Console.WriteLine("\nDigit to search: " + n);
Console.WriteLine("Check if the said digit is present in the array: " + test(nums, n));
n = "4";
Console.WriteLine("\nDigit to search: " + n);
Console.WriteLine("Check if the said digit is present in the array: " + test(nums, n));
}
// Method to check if a specified digit is present in the array
public static string test(int[] nums, string n)
{
// Using LINQ to convert each integer in the array to a string and checking if it contains the specified digit
return nums.Select(s => $"{s}").Any(a => a.Contains(n)) ? "Present" : "Not present..!";
}
}
}
Sample Output:
Original array elements: 7, 5, 85, 9, 11, 23, 18 Digit to search: 1 Check the said digit present in the array!: Present Digit to search: 9 Check the said digit present in the array!: Present Digit to search: 4 Check the said digit present in the array!: Not present..!
Flowchart:
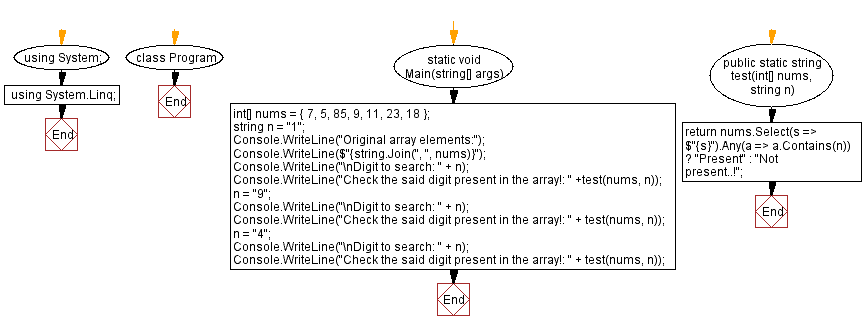
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous C# Sharp Exercise: Smallest gap between the numbers in an array.
Next C# Sharp Exercise: Sum of all prime numbers in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.