C#: Find the missing number in a given array of numbers between 10 and 20
Write a C# Sharp program to find the missing number in a given array of numbers between 10 and 20.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing an array of integers
int[] nums = { 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20 };
// Displaying original array elements
Console.WriteLine("Original array elements:");
Array.ForEach(nums, Console.WriteLine);
// Finding the missing number in the array (10-20) using the test method
Console.WriteLine("Missing number in the said array (10-20): " + test(nums));
// Creating additional arrays with missing elements and testing the 'test' method
int[] nums1 = { 11, 12, 13, 14, 15, 16, 17, 18, 19, 20 };
Console.WriteLine("\nOriginal array elements:");
Array.ForEach(nums1, Console.WriteLine);
Console.WriteLine("Missing number in the said array (10-20): " + test(nums1));
int[] nums2 = { 10, 11, 12, 13, 14, 16, 17, 18, 19, 20 };
Console.WriteLine("\nOriginal array elements:");
Array.ForEach(nums2, Console.WriteLine);
Console.WriteLine("Missing number in the said array (10-20): " + test(nums2));
int[] nums3 = { 10, 11, 12, 13, 14, 15, 16, 17, 18, 19 };
Console.WriteLine("\nOriginal array elements:");
Array.ForEach(nums3, Console.WriteLine);
Console.WriteLine("Missing number in the said array (10-20): " + test(nums3));
}
// Method to find the missing number in the array (10-20)
public static int test(int[] arr)
{
// Returning the missing number by subtracting the sum of array elements from the expected sum
return 165 - arr.Sum();
}
}
}
Sample Output:
Original array elements: 10 11 12 13 14 15 16 17 18 19 20 Missing number in the said array (10-20): 0 Original array elements: 11 12 13 14 15 16 17 18 19 20 Missing number in the said array (10-20): 10 Original array elements: 10 11 12 13 14 16 17 18 19 20 Missing number in the said array (10-20): 15 Original array elements: 10 11 12 13 14 15 16 17 18 19 Missing number in the said array (10-20): 20
Flowchart:
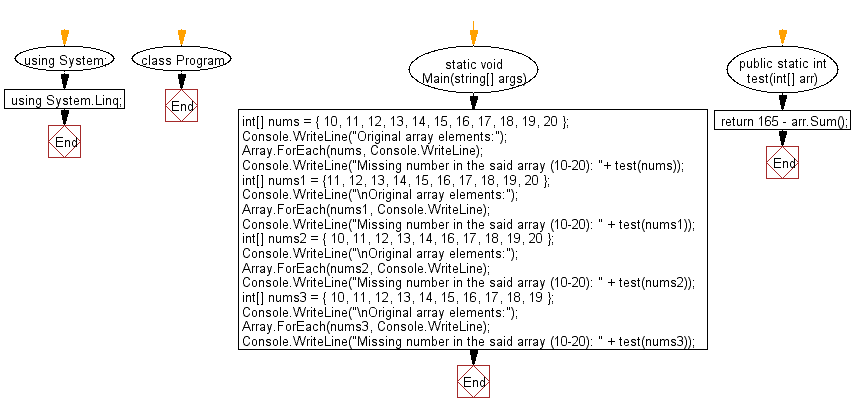
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to remove all duplicate elements from a given array and returns a new array.
Next: Write a C# Sharp program to calculate the sum of two lowest negative numbers of a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.