C#: Get only the odd values from a given array of integers
C# Sharp Array: Exercise-32 with Solution
Write a C# Sharp program to get only odd values from a given integer array.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Define an array of integers
int[] nums = { 1, 2, 3, 5, 4, 2, 3, 4 };
// Call the 'test' method to filter odd values from the 'nums' array
int[] result = test(nums);
// Display the odd values of the 'nums' array
Console.WriteLine("Only the odd values of the said array:");
Array.ForEach(result, Console.WriteLine);
// Define another array of integers
int[] nums1 = { 2, 4, 2, 6, 4, 8 };
// Call the 'test' method to filter odd values from the 'nums1' array
result = test(nums1);
// Display the odd values of the 'nums1' array
Console.WriteLine("Only the odd values of the said array:");
Array.ForEach(result, Console.WriteLine);
}
// Method to filter odd values from an integer array
public static int[] test(int[] arr)
{
// Use LINQ to filter odd values from the input array and convert the result back to an array
return arr.Where(n => n % 2 != 0).ToArray();
}
}
}
Sample Output:
Only the odd values of the said array: 1 3 5 3 Only the odd values of the said array:
Flowchart:
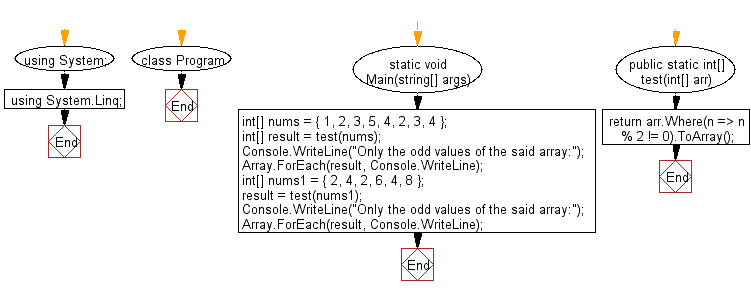
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to Check whether a Given Matrix is an Identity Matrix.
Next: Write a C# Sharp program to remove all duplicate elements from a given array and returns a new array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/array/csharp-array-exercise-32.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics