C#: Check whether a given matrix is an Identity Matrix
Write a C# Sharp program to Check whether a Given Matrix is an Identity Matrix.
Sample Solution:-
C# Sharp Code:
using System;
class Exercise31
{
public static void Main()
{
// Declaration of variables and matrix
int[,] arr1 = new int[50,50]; // Declare a matrix of maximum size 50x50 to store user input
int i, j, r1, c1; // Declare variables for iteration and matrix dimensions
// User input for square matrix order
Console.Write("\n\n Check whether a given matrix is an Identity Matrix :\n ");
Console.Write("-----------------------------------------------------------\n");
Console.Write(" Input the orders (2x2, 3x3, ...) of square matrix : ");
r1 = Convert.ToInt32(Console.ReadLine());
c1 = r1;
// User input for elements of the square matrix
Console.Write(" Input elements in the matrix :\n");
for (i = 0; i < r1; i++) // Loop through rows
{
for (j = 0; j < c1; j++) // Loop through columns
{
// Prompt user to enter matrix element
Console.Write(" element - [{0}],[{1}] : ", i, j);
arr1[i, j] = Convert.ToInt32(Console.ReadLine()); // Read user input and store in the matrix
}
}
// Display the entered matrix
Console.Write(" The matrix is :\n");
for (i = 0; i < r1; i++) // Loop through rows
{
for (j = 0; j < c1; j++) // Loop through columns
Console.Write(" {0} ", arr1[i, j]); // Print each element of the matrix
Console.Write("\n"); // Move to the next row
}
// Check if the matrix is an identity matrix
for (i = 0; i < r1; i++) // Loop through rows
{
for (j = 0; j < c1; j++) // Loop through columns
{
// Check conditions for identity matrix: diagonal elements should be 1 and non-diagonal elements should be 0
if ((i == j && arr1[i, j] != 1) || (i != j && arr1[i, j] != 0))
{
goto label; // If conditions are not met, jump to the label
}
}
}
Console.WriteLine(" The matrix is an Identity Matrix.\n\n"); // Display message if the matrix is an identity matrix
return; // Exit the program after identifying the matrix as an identity matrix
label:
Console.WriteLine("\n The matrix is not an Identity Matrix\n\n"); // Display message if the matrix is not an identity matrix
}
}
Sample Output:
Check whether a given matrix is an Identity Matrix : ----------------------------------------------------------- Input the orders(2x2, 3x3, ...) of square matrix : 2 Input elements in the matrix : element - [0],[0] : 1 element - [0],[1] : 0 element - [1],[0] : 0 element - [1],[1] : 1 The matrix is : 1 0 0 1 The matrix is an Identity Matrix
Flowchart:
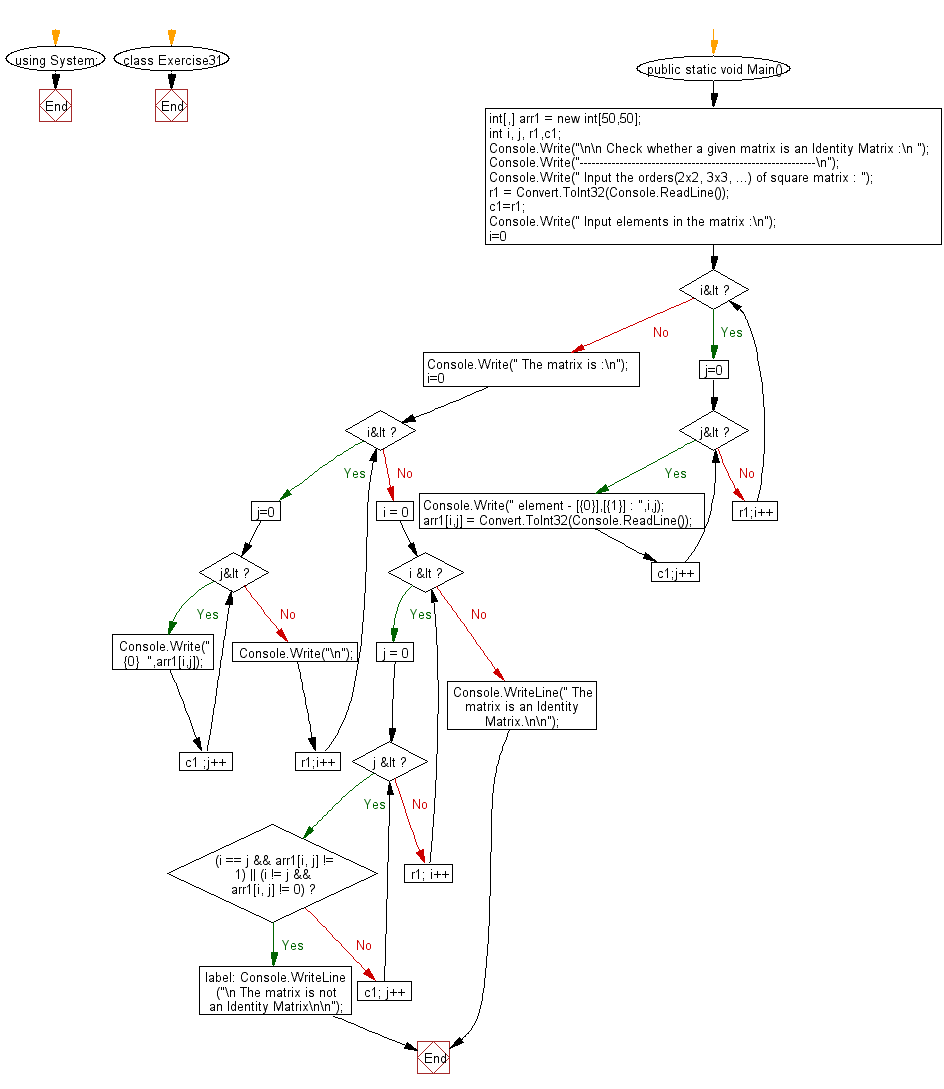
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to accept two matrices and check whether they are equal.
Next: Write a C# Sharp program to get only the odd values from a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics