C#: Accept two matrices and check whether they are equal
Write a C# Sharp program to accept two matrices and check equality.
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise30
{
public static void Main()
{
// Declaration of matrices and variables for matrix manipulation
int[,] arr1 = new int[50, 50]; // Declare the first matrix
int[,] brr1 = new int[50, 50]; // Declare the second matrix
int i, j, r1, c1, r2, c2, flag = 1; // Declare variables for iteration and matrix dimensions
// User input for dimensions of the first matrix
Console.Write("\n\nAccept two matrices and check whether they are equal :\n ");
Console.Write("-----------------------------------------------------------\n");
Console.Write("Input the number of rows in the 1st matrix : ");
r1 = Convert.ToInt32(Console.ReadLine()); // Read number of rows for the first matrix
Console.Write("Input the number of columns in the 1st matrix : ");
c1 = Convert.ToInt32(Console.ReadLine()); // Read number of columns for the first matrix
// User input for dimensions of the second matrix
Console.Write("Input the number of rows in the 2nd matrix : ");
r2 = Convert.ToInt32(Console.ReadLine()); // Read number of rows for the second matrix
Console.Write("Input the number of columns in the 2nd matrix : ");
c2 = Convert.ToInt32(Console.ReadLine()); // Read number of columns for the second matrix
// User input for elements of the first matrix
Console.Write("Input elements in the first matrix :\n");
for (i = 0; i < r1; i++) // Loop through rows of the first matrix
{
for (j = 0; j < c1; j++) // Loop through columns of the first matrix
{
// Prompt user to enter matrix element
Console.Write("element - [{0}],[{1}] : ", i, j);
arr1[i, j] = Convert.ToInt32(Console.ReadLine()); // Read user input and store in the first matrix
}
}
// User input for elements of the second matrix
Console.Write("Input elements in the second matrix :\n");
for (i = 0; i < r2; i++) // Loop through rows of the second matrix
{
for (j = 0; j < c2; j++) // Loop through columns of the second matrix
{
// Prompt user to enter matrix element
Console.Write("element - [{0}],[{1}] : ", i, j);
brr1[i, j] = Convert.ToInt32(Console.ReadLine()); // Read user input and store in the second matrix
}
}
// Display the first matrix
Console.Write("The first matrix is :\n");
for (i = 0; i < r1; i++) // Loop through rows of the first matrix
{
for (j = 0; j < c1; j++) // Loop through columns of the first matrix
Console.Write("{0} ", arr1[i, j]); // Print each element of the first matrix
Console.Write("\n"); // Move to the next row
}
// Display the second matrix
Console.Write("The second matrix is :\n");
for (i = 0; i < r2; i++) // Loop through rows of the second matrix
{
for (j = 0; j < c2; j++) // Loop through columns of the second matrix
Console.Write("{0} ", brr1[i, j]); // Print each element of the second matrix
Console.Write("\n"); // Move to the next row
}
/* Comparing two matrices for equality */
if (r1 != r2 || c1 != c2)
{
Console.Write("The Matrices Cannot be compared :\n"); // Display message if matrices have different dimensions
}
else
{
Console.Write("The Matrices can be compared : \n"); // Display message if matrices can be compared
for (i = 0; i < r1; i++) // Loop through rows
{
for (j = 0; j < c2; j++) // Loop through columns
{
if (arr1[i, j] != brr1[i, j]) // Check for inequality of corresponding elements
{
flag = 0; // Set flag to 0 if unequal elements are found
break; // Break the loop as inequality is found
}
}
}
if (flag == 1)
Console.Write("Two matrices are equal.\n\n"); // Display message if matrices are equal
else
Console.Write("But, two matrices are not equal\n\n"); // Display message if matrices are not equal
}
}
}
Sample Output:
Accept two matrices and check whether they are equal : ----------------------------------------------------------- Input the number of rows in the 1st matrix : 2 Input the number of columns in the 1st matrix : 2 Input the number of rows in the 2nd matrix : 2 Input the number of columns in the 2nd matrix : 2 Input elements in the first matrix : element - [0],[0] : 1 element - [0],[1] : 2 element - [1],[0] : 3 element - [1],[1] : 4 Input elements in the second matrix : element - [0],[0] : 5 element - [0],[1] : 6 element - [1],[0] : 7 element - [1],[1] : 8 The first matrix is : 1 2 3 4 The second matrix is : 5 6 7 8 The Matrices can be compared : But,two matrices are not equal
Flowchart:
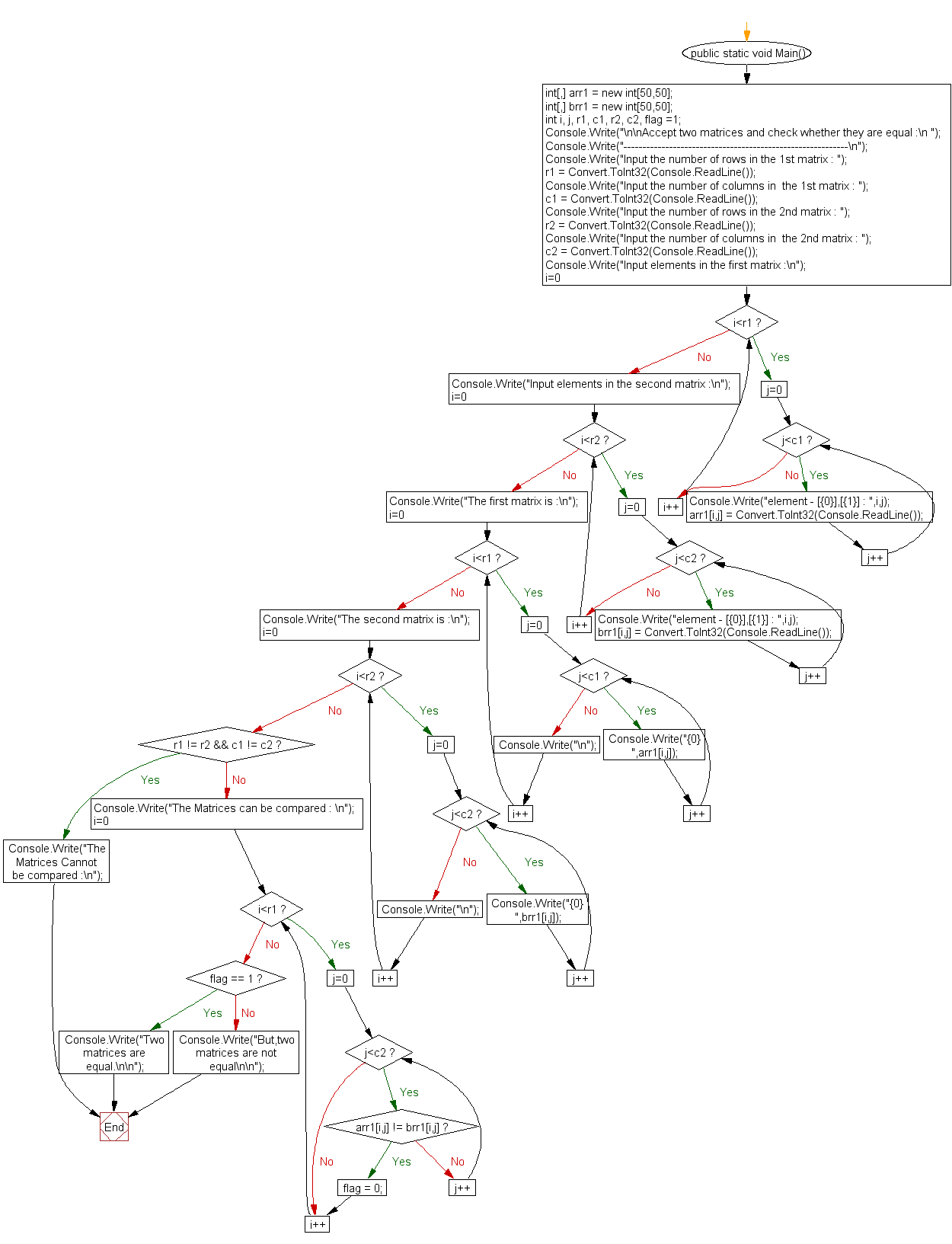
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to accept a matrix and determine whether it is a sparse matrix.
Next: Write a program in C# Sharp to Check whether a Given Matrix is an Identity Matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.