C#: Display the upper triangular of a given matrix
Write a C# Sharp program to print or display an upper triangular matrix.
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise27
{
public static void Main()
{
int i, j, n;
int[,] arr1 = new int[50, 50];
// Prompt user for the size of the square matrix
Console.Write("\n\nDisplay the upper triangular of a given matrix :\n");
Console.Write("----------------------------------------------\n");
Console.Write("Input the size of the square matrix : ");
n = Convert.ToInt32(Console.ReadLine());
// Input elements into the matrix
Console.Write("Input elements in the first matrix :\n");
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
{
Console.Write("element - [{0}],[{1}] : ", i, j);
arr1[i, j] = Convert.ToInt32(Console.ReadLine());
}
}
// Display the input matrix
Console.Write("The matrix is :\n");
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
Console.Write("{0} ", arr1[i, j]);
Console.Write("\n");
}
// Display the upper triangular matrix by setting non-upper triangular elements to zero
Console.Write("\nSetting zero in upper triangular matrix\n");
for (i = 0; i < n; i++)
{
Console.Write("\n");
for (j = 0; j < n; j++)
{
if (i >= j)
Console.Write("{0} ", arr1[i, j]); // Display upper triangular elements
else
Console.Write("{0} ", 0); // Display zero for non-upper triangular elements
}
}
Console.Write("\n\n");
}
}
Sample Output:
Display the upper triangular of a given matrix : ---------------------------------------------- Input the size of the square matrix : 3 Input elements in the first matrix : element - [0],[0] : 1 element - [0],[1] : 2 element - [0],[2] : 3 element - [1],[0] : 4 element - [1],[1] : 5 element - [1],[2] : 6 element - [2],[0] : 7 element - [2],[1] : 8 element - [2],[2] : 9 The matrix is : 1 2 3 4 5 6 7 8 9 Setting zero in upper triangular matrix 1 0 0 4 5 0 7 8 9
Flowchart:
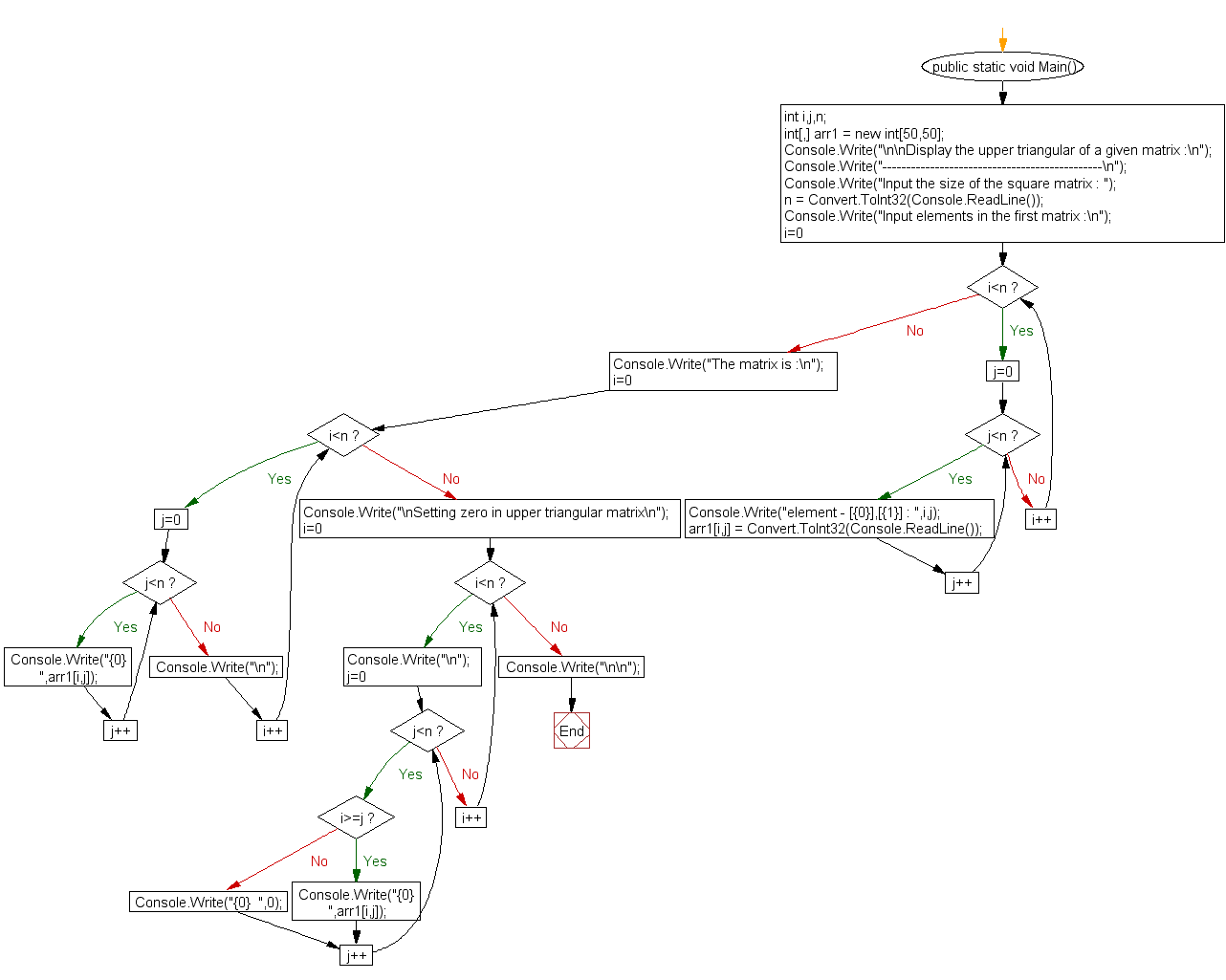
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to print or display the lower triangular of a given matrix.
Next: Write a program in C# Sharp to calculate determinant of a 3 x 3 matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics