C#: Display the lower triangular of a given matrix
Write a program in C# Sharp to print or display the lower triangular of a given matrix.
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise26
{
public static void Main()
{
int i, j, n; // Declare variables for iteration and matrix size
int[,] arr1 = new int[10, 10]; // Declare a 2D array to store the matrix
Console.Write("\n\nDisplay the lower triangular of a given matrix :\n");
Console.Write("----------------------------------------------------\n");
Console.Write("Input the size of the square matrix : ");
n = Convert.ToInt32(Console.ReadLine()); // Input the size of the square matrix
Console.Write("Input elements in the matrix :\n");
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
{
Console.Write("element - [{0}],[{1}] : ", i, j);
arr1[i, j] = Convert.ToInt32(Console.ReadLine()); // Input matrix elements
}
}
Console.Write("The matrix is :\n");
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
{
Console.Write("{0} ", arr1[i, j]); // Display the original matrix
}
Console.Write("\n");
}
Console.Write("\nSetting zero in lower triangular matrix\n");
for (i = 0; i < n; i++)
{
Console.Write("\n");
for (j = 0; j < n; j++)
{
if (i <= j)
{
Console.Write("{0} ", arr1[i, j]); // Display elements in the lower triangular part
}
else
{
Console.Write("{0} ", 0); // Display zeros in the upper triangular part
}
}
}
Console.Write("\n\n");
}
}
Sample Output:
Display the lower triangular of a given matrix : ---------------------------------------------------- Input the size of the square matrix : 3 Input elements in the first matrix : element - [0],[0] : 1 element - [0],[1] : 2 element - [0],[2] : 3 element - [1],[0] : 4 element - [1],[1] : 5 element - [1],[2] : 6 element - [2],[0] : 7 element - [2],[1] : 8 element - [2],[2] : 9 The matrix is : 1 2 3 4 5 6 7 8 9 Setting zero in lower triangular matrix 1 2 3 0 5 6 0 0 9
Flowchart:
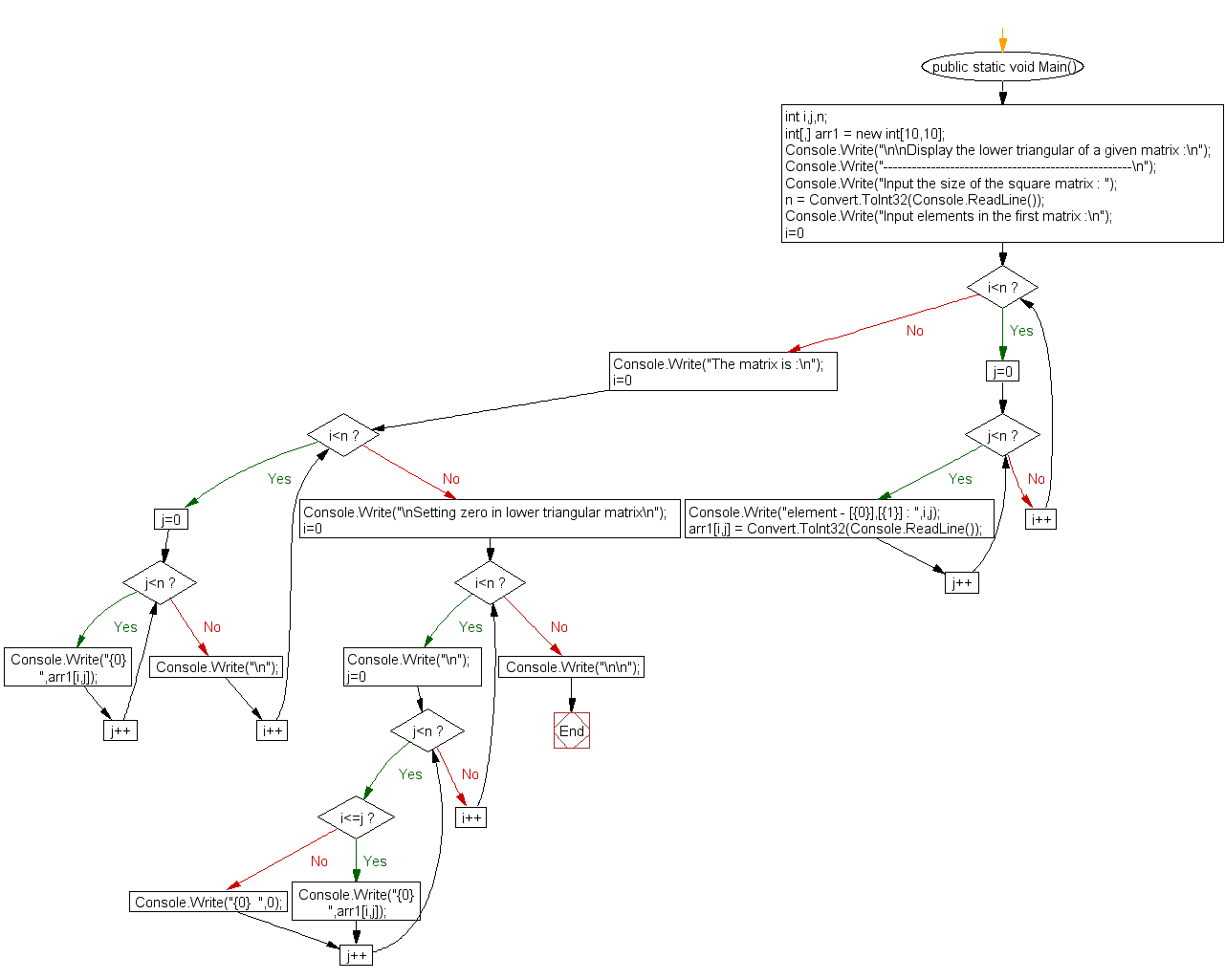
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find the sum of rows an columns of a Matrix.
Next: Write a program in C# Sharp to print or display upper triangular matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.