C#: Find the sum of left diagonals of a matrix
Write a C# Sharp program to find the sum of the left diagonals of a matrix.
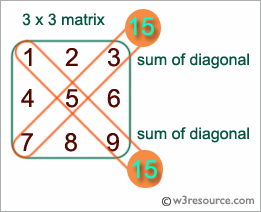
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise24
{
public static void Main()
{
int i, j, sum = 0, n, m = 0; // Declare variables for iteration, sum, matrix size, and auxiliary variable m
int[,] arr1 = new int[50, 50]; // Declare the matrix
Console.Write("\n\nFind the sum of left diagonals of a matrix :\n");
Console.Write("--------------------------------------------\n");
Console.Write("Input the size of the square matrix : ");
n = Convert.ToInt32(Console.ReadLine()); // Input the size of the square matrix
m = n; // Store the size of the matrix in the auxiliary variable m
Console.Write("Input elements in the matrix :\n");
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
{
Console.Write("element - [{0}],[{1}] : ", i, j);
arr1[i, j] = Convert.ToInt32(Console.ReadLine()); // Input matrix elements
}
}
Console.Write("The matrix is :\n");
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
{
Console.Write("{0} ", arr1[i, j]); // Display the matrix
}
Console.Write("\n");
}
// Calculate the sum of left diagonals
for (i = 0; i < n; i++)
{
m = m - 1; // Decrease m for each row iteration
for (j = 0; j < n; j++)
{
if (j == m)
{
sum += arr1[i, j]; // Accumulate the sum of left diagonal elements
}
}
}
Console.Write("Addition of the left Diagonal elements is : {0}\n", sum); // Display sum of left diagonal elements
}
}
Sample Output:
Find the sum of left diagonals of a matrix : -------------------------------------------- Input the size of the square matrix : 2 Input elements in the matrix : element - [0],[0] : 2 element - [0],[1] : 4 element - [1],[0] : 5 element - [1],[1] : 6 The matrix is : 2 4 5 6 Addition of the left Diagonal elements is :9
Flowchart:
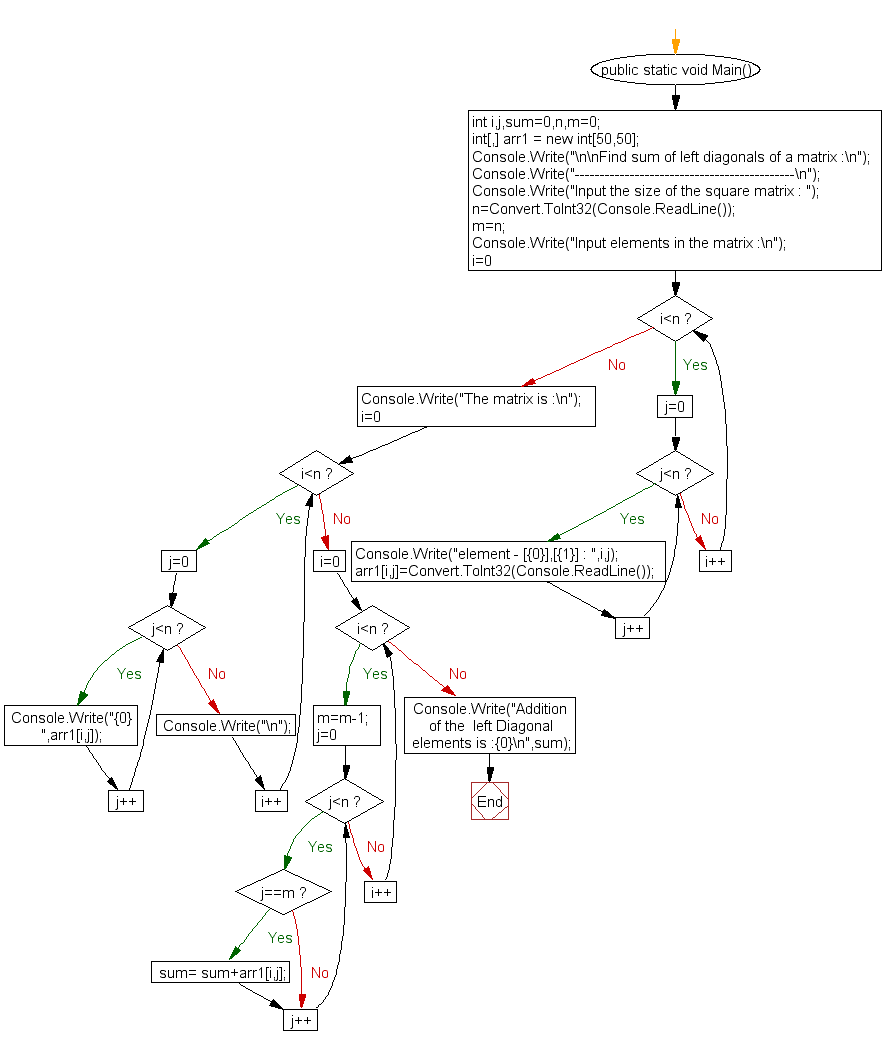
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find sum of right diagonals of a matrix.
Next: Write a program in C# Sharp to find the sum of rows an columns of a Matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.