C#: Find sum of right diagonals of a matrix
C# Sharp Array: Exercise-23 with Solution
Write a C# Sharp program to find the sum of the right diagonals of a matrix.
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise23
{
public static void Main()
{
int i, j, sum = 0, n; // Declare variables for iteration, sum, and matrix size
int[,] arr1 = new int[50, 50]; // Declare the matrix
Console.Write("\n\nFind sum of right diagonals of a matrix :\n");
Console.Write("---------------------------------------\n");
Console.Write("Input the size of the square matrix : ");
n = Convert.ToInt32(Console.ReadLine()); // Input the size of the square matrix
Console.Write("Input elements in the matrix :\n");
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
{
Console.Write("element - [{0}],[{1}] : ", i, j);
arr1[i, j] = Convert.ToInt32(Console.ReadLine()); // Input matrix elements
if (i == j) // Check if it's the right diagonal (i.e., where row index equals column index)
{
sum += arr1[i, j]; // Accumulate the sum of the right diagonal elements
}
}
}
Console.Write("The matrix is :\n");
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
{
Console.Write("{0} ", arr1[i, j]); // Display the matrix
}
Console.Write("\n");
}
Console.Write("Addition of the right Diagonal elements is : {0}\n", sum); // Display sum of right diagonal elements
}
}
Sample Output:
Find sum of right diagonals of a matrix : --------------------------------------- Input the size of the square matrix : 3 Input elements in the first matrix : element - [0],[0] : 1 element - [0],[1] : 2 element - [0],[2] : 3 element - [1],[0] : 4 element - [1],[1] : 5 element - [1],[2] : 6 element - [2],[0] : 7 element - [2],[1] : 8 element - [2],[2] : 9 The matrix is : 1 2 3 4 5 6 7 8 9 Addition of the right Diagonal elements is :15
Flowchart:
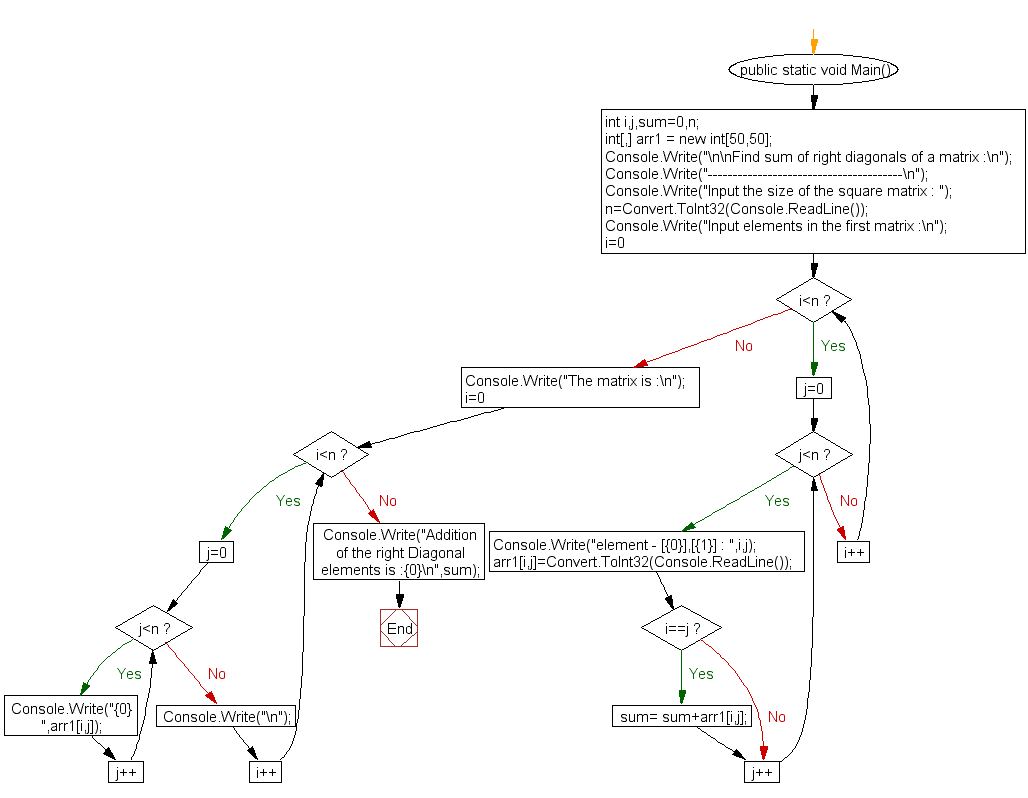
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find transpose of a given matrix.
Next: Write a program in C# Sharp to find sum of left diagonals of a matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/array/csharp-array-exercise-23.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics