C#: Transpose of a specified Matrix
Write a C# Sharp program to find the transpose of a given matrix.
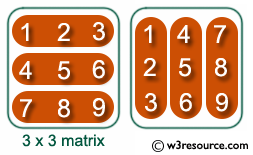
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise22
{
public static void Main()
{
int i, j, r, c;
int[,] arr1 = new int[50, 50]; // Declare the first matrix
int[,] brr1 = new int[50, 50]; // Declare the transpose matrix
Console.Write("\n\nTranspose of a Matrix :\n");
Console.Write("---------------------------\n");
Console.Write("\nInput the number of rows and columns of the matrix :\n");
Console.Write("Rows : ");
r = Convert.ToInt32(Console.ReadLine());
Console.Write("Columns : ");
c = Convert.ToInt32(Console.ReadLine());
// Input elements into the matrix
Console.Write("Input elements in the matrix :\n");
for (i = 0; i < r; i++)
{
for (j = 0; j < c; j++)
{
Console.Write("element - [{0}],[{1}] : ", i, j);
arr1[i, j] = Convert.ToInt32(Console.ReadLine());
}
}
// Display the original matrix
Console.Write("\nThe Original matrix is :\n");
for (i = 0; i < r; i++)
{
Console.Write("\n");
for (j = 0; j < c; j++)
Console.Write("{0}\t", arr1[i, j]);
}
// Transpose the matrix
for (i = 0; i < r; i++)
{
for (j = 0; j < c; j++)
{
brr1[j, i] = arr1[i, j]; // Swap rows and columns for the transpose
}
}
// Display the transpose of the matrix
Console.Write("\n\nThe Transpose of the matrix is : ");
for (i = 0; i < c; i++)
{
Console.Write("\n");
for (j = 0; j < r; j++)
{
Console.Write("{0}\t", brr1[i, j]);
}
}
Console.Write("\n\n");
}
}
Sample Output:
Transpose of a Matrix : --------------------------- Input the number of rows and columns of the first matrix : Rows : 2 Columns : 2 Input elements in the matrix : element - [0],[0] : 1 element - [0],[1] : 2 element - [1],[0] : 3 element - [1],[1] : 4 The First matrix is : 1 2 3 4 The Transpose of a matrix is : 1 3 2 4
Flowchart:
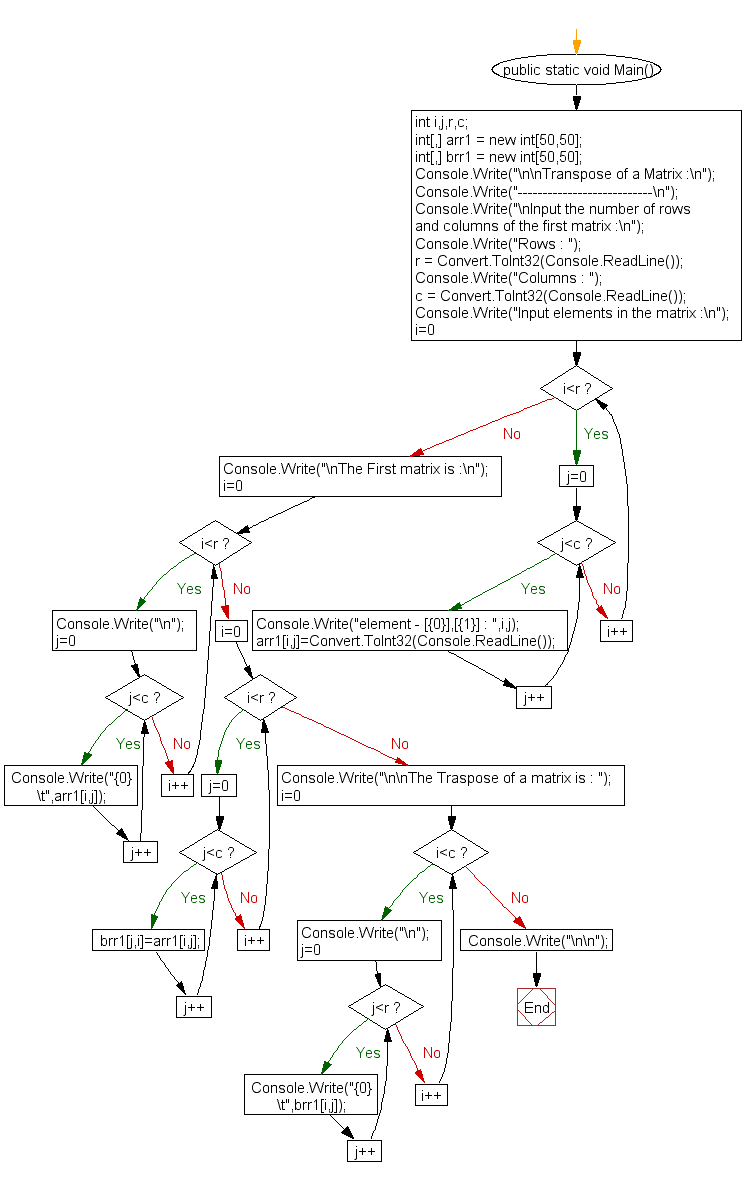
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp for multiplication of two square Matrices.
Next: Write a program in C# Sharp to find sum of right diagonals of a matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.