C#: Read n number of values in an array and display it in reverse order
Write a C# Sharp program to read n values in an array and display them in reverse order.
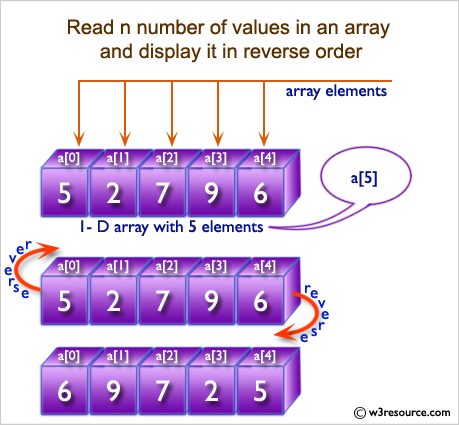
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise2 // Declaration of the Exercise2 class
{
public static void Main() // Main method, entry point of the program
{
int i, n; // Declaration of variables 'i' and 'n'
int[] a = new int[100]; // Declaration of an integer array 'a' with size 100
// Display a message about reading n number of values in an array and displaying it in reverse order
Console.Write("\n\nRead n number of values in an array and display it in reverse order:\n");
Console.Write("------------------------------------------------------------------------\n");
Console.Write("Input the number of elements to store in the array: "); // Prompt the user to input the number of elements
n = Convert.ToInt32(Console.ReadLine()); // Read the number of elements from the user and store it in 'n'
Console.Write("Input {0} number of elements in the array:\n", n); // Prompt the user to input 'n' elements
// Loop to read 'n' elements from the user and store them in the array 'a'
for (i = 0; i < n; i++)
{
Console.Write("element - {0} : ", i); // Prompt for input element number
a[i] = Convert.ToInt32(Console.ReadLine()); // Read user input and store it in the array 'a'
}
Console.Write("\nThe values stored into the array are:\n");
// Loop to display the elements stored in the array 'a'
for (i = 0; i < n; i++)
{
Console.Write("{0} ", a[i]); // Display each element of the array 'a'
}
Console.Write("\n\nThe values stored into the array in reverse are:\n");
// Loop to display the elements stored in the array 'a' in reverse order
for (i = n - 1; i >= 0; i--)
{
Console.Write("{0} ", a[i]); // Display each element of the array 'a' in reverse order
}
Console.Write("\n\n"); // Move to the next line for better readability
}
}
Sample Output:
Read n number of values in an array and display it in reverse order: ------------------------------------------------------------------------ Input the number of elements to store in the array :3 Input 3 number of elements in the array : element - 0 : 1 element - 1 : 2 element - 2 : 3 The values store into the array are : 1 2 3 The values store into the array in reverse are : 3 2 1
Flowchart:
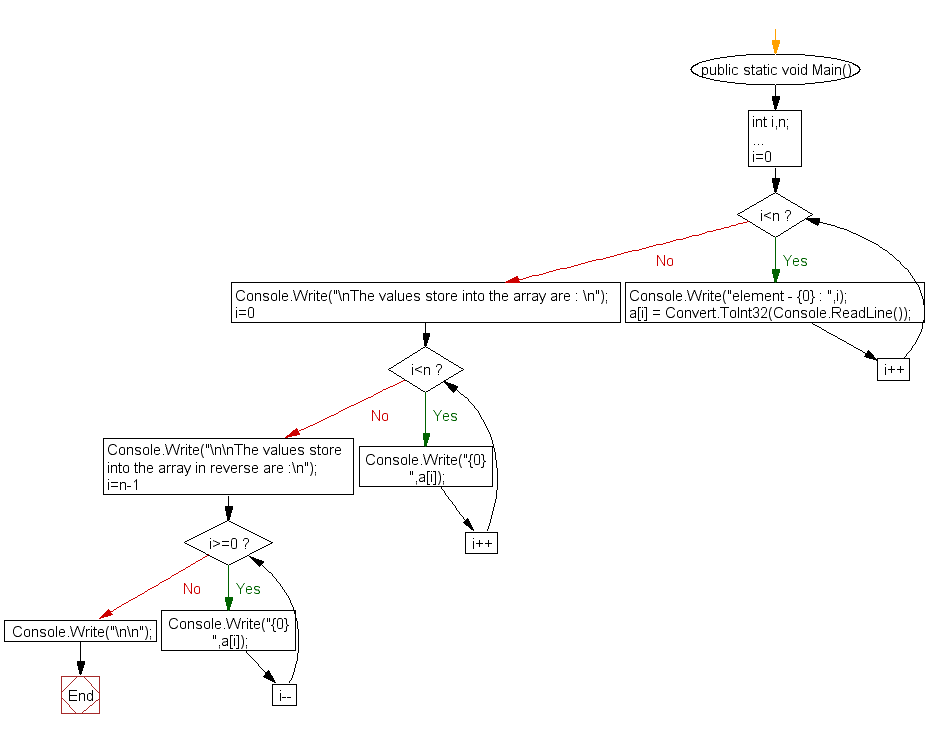
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to store elements in an array and print it.
Next: Write a program in C# Sharp to find the sum of all elements of array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics