C#: Find the second smallest element in an array
Write a C# Sharp program to find the second smallest element in an array.
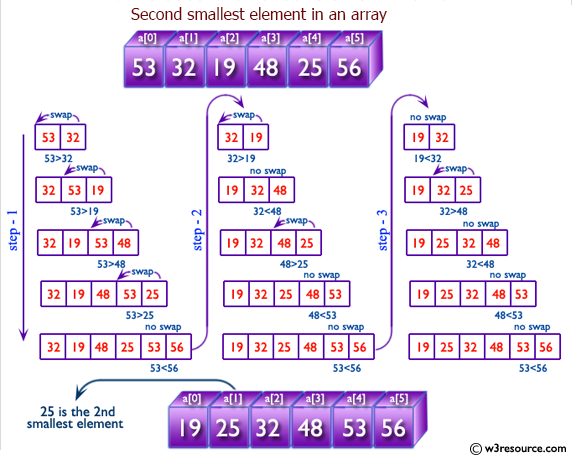
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise17
{
public static void Main()
{
int n, i, j = 0, sml, sml2nd; // Declare variables for array size, counting, and finding smallest elements
int[] arr1 = new int[50]; // Declare an array to store integers
// Display a message prompting the user to input the size of the array
Console.Write("\n\nFind the second smallest element in an array :\n");
Console.Write("-----------------------------------------\n");
Console.Write("Input the size of array : ");
n = Convert.ToInt32(Console.ReadLine()); // Read the size of the array entered by the user
/* Store values into the array */
Console.Write("Input {0} elements in the array (value must be <9999):\n", n);
for (i = 0; i < n; i++)
{
Console.Write("element - {0} : ", i);
arr1[i] = Convert.ToInt32(Console.ReadLine()); // Store user input in the array
}
/* Find the location of the smallest element in the array */
sml = 99999; // Initialize the smallest element with a large value to ensure comparison
for (i = 0; i < n; i++)
{
if (sml > arr1[i])
{
sml = arr1[i]; // Find the smallest element
j = i; // Store the index of the smallest element
}
}
/* Find the second smallest element in the array by ignoring the smallest element */
sml2nd = 99999;
for (i = 0; i < n; i++)
{
if (i == j)
{
i++; /* Ignore the smallest element */
i--;
}
else
{
if (sml2nd > arr1[i])
{
sml2nd = arr1[i]; // Find the second smallest element
}
}
}
Console.Write("The Second smallest element in the array is : {0} \n\n", sml2nd); // Display the second smallest element
}
}
Sample Output:
Find the second smallest element in an array : ----------------------------------------- Input the size of array : 5 Input 5 elements in the array (value must be <9999>: element - 0 : 2 element - 1 : 4 element - 2 : 6 element - 3 : 8 element - 4 : 10 The Second smallest element in the array is : 4
Flowchart:
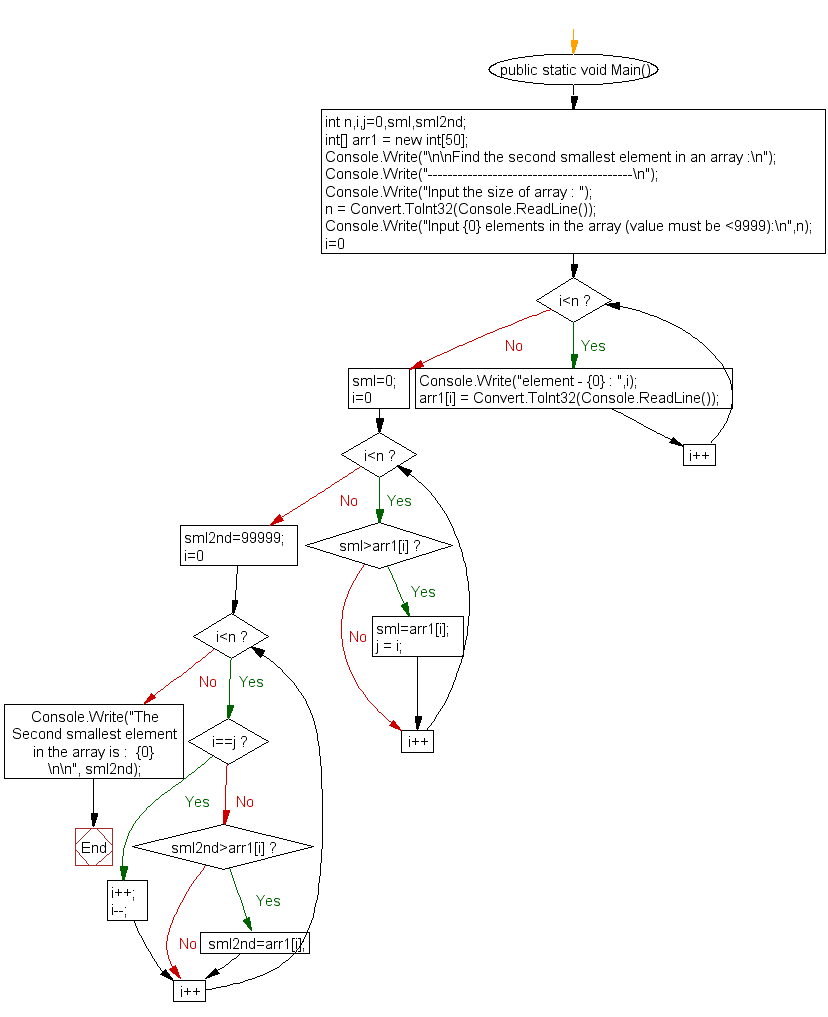
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find the second largest element in an array.
Next: Write a program in C# Sharp for a 2D array of size 3x3 and print the matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.