C#: Delete an element at desired position from an array
Write a C# Sharp program to delete an element at the desired position from an array.
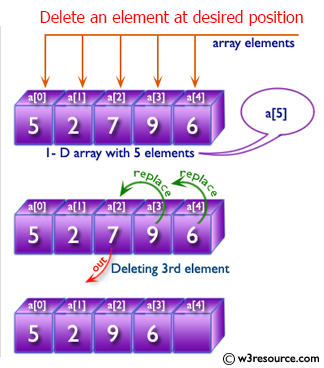
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise15
{
public static void Main()
{
int i, pos, n; // Declare variables for counting, position, and array size
int[] arr1 = new int[50]; // Declare an array to store integers
// Display a message prompting the user to input the size of the array
Console.Write("\n\nDelete an element at desired position from an array :\n");
Console.Write("---------------------------------------------------------\n");
Console.Write("Input the size of array : ");
n = Convert.ToInt32(Console.ReadLine()); // Read the size of the array entered by the user
/* Store values into the array */
Console.Write("Input {0} elements in the array in ascending order:\n", n);
for (i = 0; i < n; i++)
{
Console.Write("element - {0} : ", i);
arr1[i] = Convert.ToInt32(Console.ReadLine()); // Store user input in the array
}
Console.Write("\nInput the position where to delete: ");
pos = Convert.ToInt32(Console.ReadLine()); // Read the position of the element to be deleted
/* Locate the position in the array */
i = 0;
while (i != pos - 1)
i++; // Find the index position that needs to be deleted
/* Replace the element at the position with its right neighbor */
while (i < n)
{
arr1[i] = arr1[i + 1]; // Shift elements to the left to overwrite the element to be deleted
i++;
}
n--; // Decrease the size of the array by one after deletion
Console.Write("\nThe new list is : ");
for (i = 0; i < n; i++)
{
Console.Write(" {0}", arr1[i]); // Display the updated array after deletion
}
Console.Write("\n\n");
}
}
Sample Output:
Delete an element at desired position from an array : --------------------------------------------------------- Input the size of array : 3 Input 3 elements in the array in ascending order: element - 0 : 2 element - 1 : 4 element - 2 : 6 Input the position where to delete: 2 The new list is : 2 6
Flowchart:
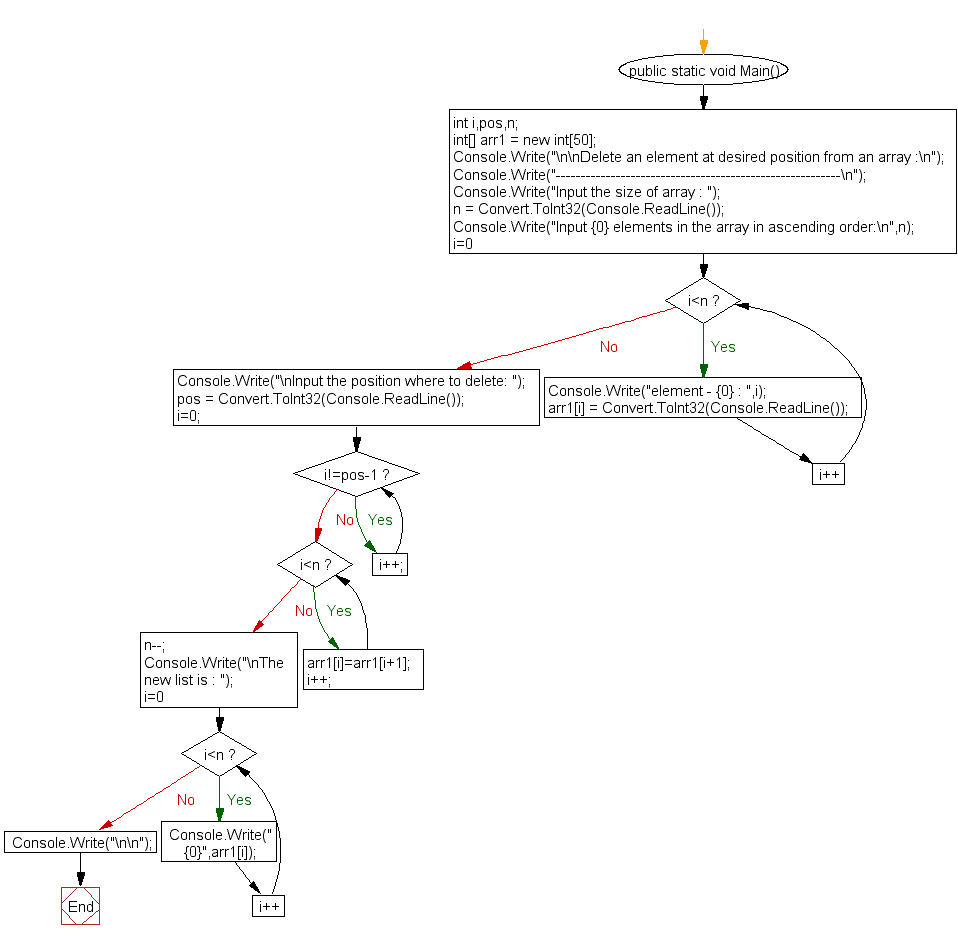
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to insert New value in the array (unsorted list ).
Next: Write a program in C# Sharp to find the second largest element in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.