C#: Read and Print elements of an array
Write a C# Sharp program that stores elements in an array and prints them.
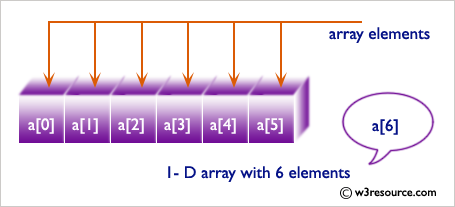
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise1 // Declaration of the Exercise1 class
{
public static void Main() // Main method, entry point of the program
{
int[] arr = new int[10]; // Declaration of an integer array with size 10
int i; // Declaration of loop variable 'i'
// Display a message about reading and printing elements of an array
Console.Write("\n\nRead and Print elements of an array:\n");
Console.Write("-----------------------------------------\n");
Console.Write("Input 10 elements in the array :\n"); // Prompt user to input 10 elements
// Loop to read 10 elements from the user and store them in the array
for(i = 0; i < 10; i++)
{
Console.Write("element - {0} : ", i); // Prompt for input element number
arr[i] = Convert.ToInt32(Console.ReadLine()); // Read user input and convert it to an integer, then store in the array
}
Console.Write("\nElements in array are: "); // Display message before printing array elements
// Loop to print the elements of the array
for(i = 0; i < 10; i++)
{
Console.Write("{0} ", arr[i]); // Print each element of the array
}
Console.Write("\n"); // Move to the next line for better readability
}
}
Sample Output:
Read and Print elements of an array: ----------------------------------------- Input 10 elements in the array : element - 0 : 2 element - 1 : 4 element - 2 : 6 element - 3 : 8 element - 4 : 10 element - 5 : 12 element - 6 : 14 element - 7 : 16 element - 8 : 18 element - 9 : 20 Elements in array are: 2 4 6 8 10 12 14 16 18 20
Flowchart:
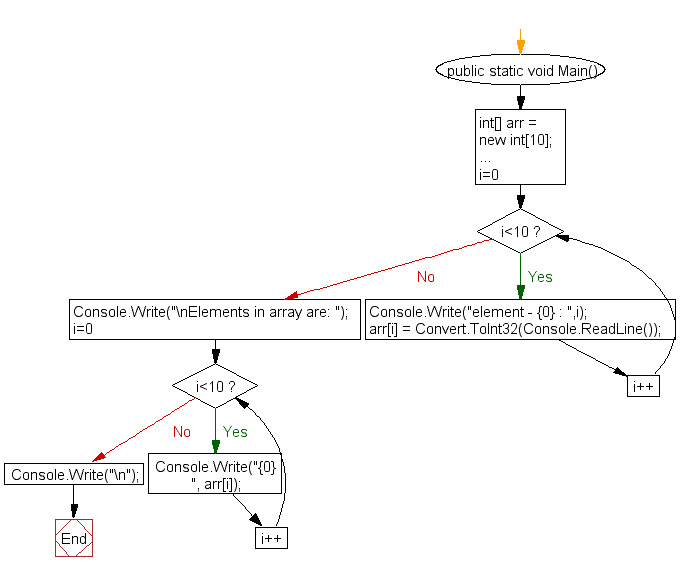
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: C# Sharp Array Exercises Home.
Next: Write a program in C# Sharp to read n number of values in an array and display it in reverse order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics