C++ Vector Exercises: Find strings that contain a number(s) from a vector
6. Filter Strings Containing Numbers from a Vector
Write a C++ program that takes a vector of strings and returns only those strings that contain a number(s). Return an empty vector if none.
Sample Data:
({"red", "green23", "1black", "white"}) -> ("green23", "1black")
({"red", "green", "black", "white"}) -> ( )
Sample Solution-1:
C++ Code:
#include <algorithm> // Including the Algorithm Library for certain functions like find_if
#include <iostream> // Including the Input/Output Stream Library
#include <vector> // Including the Vector Library for using vectors
using namespace std; // Using the Standard Namespace
// Function to find strings in the vector that contain at least one digit
std::vector<std::string> test(std::vector<std::string> colors) {
std::vector<std::string> result; // Creating a new vector to store strings containing digits
// Loop through each string in the input vector 'colors'
for(auto& text : colors){
auto it = std::find_if(text.begin(), text.end(), [](char c){return std::isdigit(c);}); // Using find_if to search for a digit in the current string
if(it != text.end()){ // If a digit is found in the string
result.push_back(text); // Add the string to the 'result' vector
}
}
return result; // Return the vector containing strings with digits
}
// Main function
int main(){
vector<string> colors = {"red", "green23", "1black", "white"}; // Initializing a vector of strings
cout << "Original Vector elements:\n";
for (string c : colors)
cout << c << " "; // Printing the original elements of the vector
vector<string> result = test(colors); // Calling the test function to find strings containing digits
cout << "\n\nFind strings that contain a number(s) from the said vector:\n";
for (string c : result)
cout << c << " "; // Printing the strings containing digits from the 'result' vector
}
Sample Output:
Original Vector elements: red green23 1black white Find strings that contain a number(s) from the said vector: green23 1black
Flowchart:
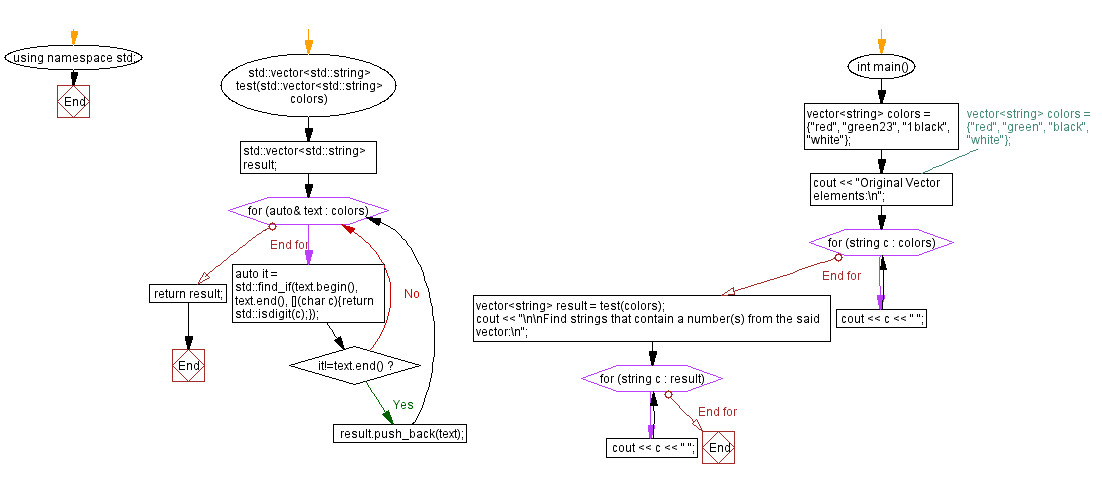
Sample Solution-2:
C++ Code:
#include <algorithm> // Including the Algorithm Library for certain functions like isdigit
#include <iostream> // Including the Input/Output Stream Library
#include <vector> // Including the Vector Library for using vectors
using namespace std; // Using the Standard Namespace
// Function to find strings in the vector that contain at least one digit
std::vector<std::string> test(std::vector<std::string> colors) {
vector<string> result; // Creating a new vector to store strings containing digits
// Loop through each string in the input vector 'colors'
for(string i : colors) {
// Loop through each character in the string
for(char el : i) {
if(isdigit(el)) { // If a digit is found in the string
result.push_back(i); // Add the string to the 'result' vector
break; // Break the loop as soon as a digit is found in the string
}
}
}
return result; // Return the vector containing strings with digits
}
// Main function
int main() {
vector<string> colors = {"red", "green23", "1black", "white"}; // Initializing a vector of strings
cout << "Original Vector elements:\n";
for (string c : colors)
cout << c << " "; // Printing the original elements of the vector
vector<string> result = test(colors); // Calling the test function to find strings containing digits
cout << "\n\nFind strings that contain a number(s) from the said vector:\n";
for (string c : result)
cout << c << " "; // Printing the strings containing digits from the 'result' vector
}
Sample Output:
Original Vector elements:
red green23 1black white
Find strings that contain a number(s) from the said vector:
green23 1black
For more Practice: Solve these Related Problems:
- Write a C++ program to iterate through a vector of strings and return a new vector containing only those strings that include at least one digit.
- Write a C++ program that reads a vector of strings and uses regular expressions to filter out strings containing numeric characters.
- Write a C++ program to scan a vector of strings and check each string for the presence of digits, then output all matching strings.
- Write a C++ program that processes a vector of strings and returns a vector of strings that contain one or more digits using character checking.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: First string contains all letters from second string.
What is the difficulty level of this exercise?