C++ Vector Exercises: First string contains all letters from second string
5. Verify Letters of Second String in First String
Write a C++ program to verify that all of the letters in the second string appear in the first string as well. Return true otherwise false.
Sample Data:
({"Python", "Py"}) -> 1
({"CPP", "C++"}) ->.0
Sample Solution-1:
C++ Code:
#include <iostream> // Including the Input/Output Stream Library
#include <vector> // Including the Vector Library for using vectors
using namespace std; // Using the Standard Namespace
// Function to check if all characters in the second string are present in the first string
string test(vector<string> strs) {
for (auto ch : strs.at(1)) { // Iterating through each character in the second string
// Checking if the uppercase or lowercase form of the character exists in the first string
if (strs.at(0).find(toupper(ch)) == string::npos && strs.at(0).find(tolower(ch)) == string::npos)
return "false"; // If a character is not found in the first string, return "false"
}
return "true"; // If all characters are found in the first string, return "true"
}
// Main function
int main() {
vector<string> strs = {"Python", "Py"}; // Initializing a vector of strings
cout << "Original String elements: ";
for (string c : strs)
cout << c << " "; // Printing the original elements of the vector
cout << "\nCheck - First string contains all letters from second string: ";
string result = test(strs); // Calling the test function to check character presence in the strings
cout << result << " "; // Printing the result ("true" or "false")
}
Sample Output:
Original String elements: Python Py Check - First string contains all letters from second string: true
Flowchart:
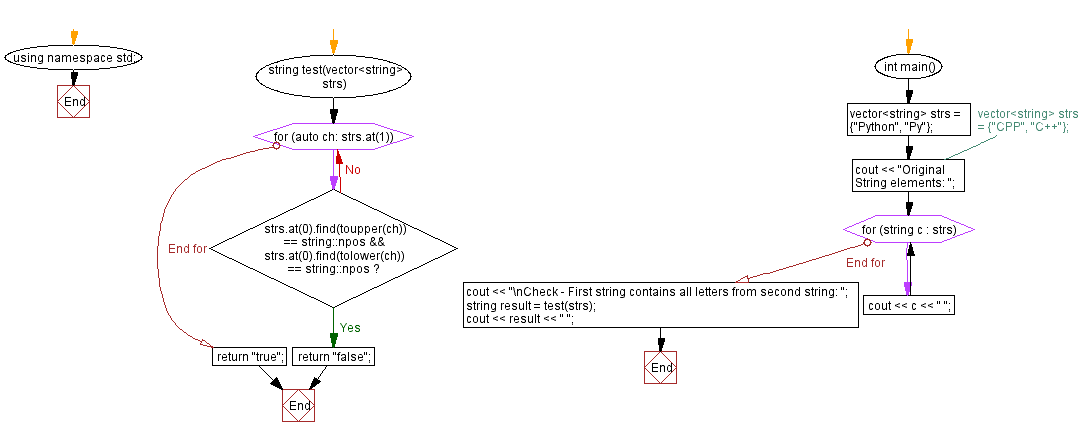
Sample Solution-2:
C++ Code:
#include <iostream> // Including the Input/Output Stream Library
#include <vector> // Including the Vector Library for using vectors
using namespace std; // Using the Standard Namespace
// Function to check if all characters in the second string are present in the first string
string test(vector<string> strs) {
// Convert all characters in the first string to uppercase
for (char &ch : strs.at(0))
ch = toupper(ch);
// Convert all characters in the second string to uppercase and check if each character exists in the first string
for (char &ch : strs.at(1)) {
ch = toupper(ch); // Convert the character to uppercase
if (strs.at(0).find(ch) == std::string::npos) // Check if the character is not found in the first string
return "false"; // If a character is not found in the first string, return "false"
}
return "true"; // If all characters are found in the first string, return "true"
}
// Main function
int main() {
// vector<string> strs = {"Python", "Py"};
vector<string> strs = {"CPP", "C++"}; // Initializing a vector of strings
cout << "Original String elements: ";
for (string c : strs)
cout << c << " "; // Printing the original elements of the vector
cout << "\nCheck - First string contains all letters from second string: ";
string result = test(strs); // Calling the test function to check character presence in the strings
cout << result << " "; // Printing the result ("true" or "false")
}
Sample Output:
Original String elements: CPP C++ Check - First string contains all letters from second string: false
Flowchart:
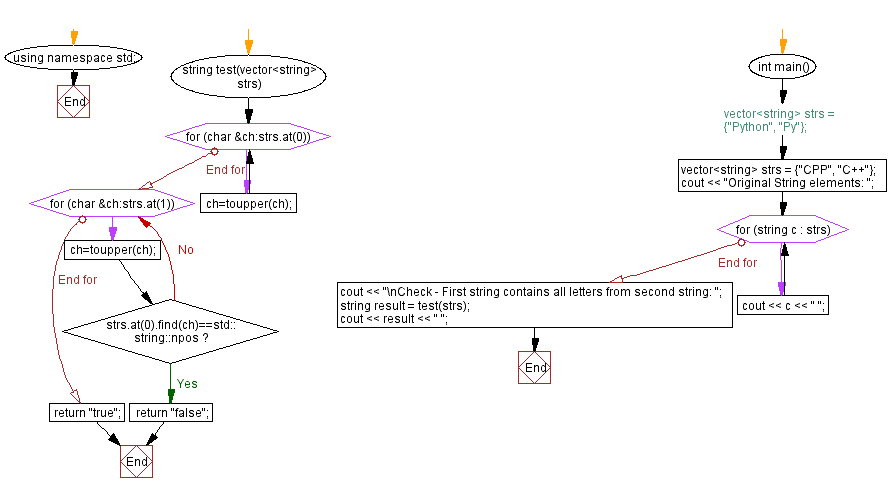
For more Practice: Solve these Related Problems:
- Write a C++ program that checks if every character from a second string is present in a first string, using a frequency array for letters.
- Write a C++ program to determine if the characters in the second string form a subsequence of the first string, preserving order.
- Write a C++ program that uses a hash set to verify that all letters of the second string appear in the first string regardless of order.
- Write a C++ program to compare two strings and return true if the first string contains all the characters (case-sensitive) of the second string.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Capitalize the first character of each vector element.
Next C++ Exercise: Find strings that contain a number(s) from a vector.
What is the difficulty level of this exercise?