C++ Vector Exercises: Vector elements smaller than its adjacent neighbours
Write a C++ program that returns the elements in a vector that are strictly smaller than their adjacent left and right neighbours.
Sample Data:
({7, 2 ,5, 3, 1, 5, 6}) -> 2, 1
({1, 2 ,5, 0, 3, 1, 7}) -> 0, 1
Sample Solution:
C++ Code:
#include <algorithm> // Including the Algorithm Library for certain functions like sort
#include <iostream> // Including the Input/Output Stream Library
#include <vector> // Including the Vector Library for using vectors
using namespace std; // Using the Standard Namespace
// Function to find elements in the vector that are smaller than both adjacent neighbors
std::vector<int> test(std::vector<int> nums)
{
std::vector<int> temp; // Initializing an empty vector to store elements that meet the condition
for(int i=1; i<nums.size()-1; i++) {
// Checking if the current element is smaller than both its previous and next elements
if(nums[i] < nums[i-1] && nums[i] < nums[i+1])
temp.push_back(nums[i]); // If the condition is met, adding the element to the 'temp' vector
}
return temp; // Returning the vector containing elements smaller than their adjacent neighbors
}
// Main function
int main(){
// Uncomment either of the following lines to test different sets of numbers
// vector<int> nums = {7, 2 ,5, 3, 1, 5, 6}; // Test vector with non-matching elements
vector<int> nums = {1, 2 ,5, 0, 3, 1, 7}; // Test vector with elements smaller than both adjacent neighbors
cout << "Original Vector elements:\n";
for (int x : nums)
cout << x << " "; // Printing the elements of the original vector
vector<int> result = test(nums); // Calling the test function to find elements smaller than both neighbors
cout << "\nVector elements that are smaller than its adjacent neighbors:\n";
for (int y : result)
cout << y << "\n"; // Printing the elements that meet the condition from the 'result' vector
}
Sample Output:
Original Vector elements: 1 2 5 0 3 1 7 Vector elements that are smaller than its adjacent neighbors: 0 1
Flowchart:
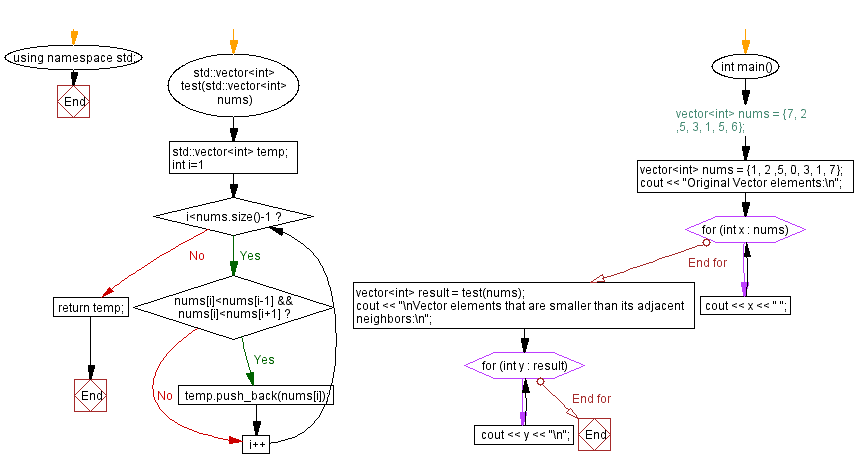
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Consecutive Numbers in a vector
Next C++ Exercise: Create an n x n matrix by taking an integer (n).
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics