C++ String Exercises: Count all the words in a given string
C++ String: Exercise-8 with Solution
Write a C++ program to count all the words in a given string.
Visual Presentation:
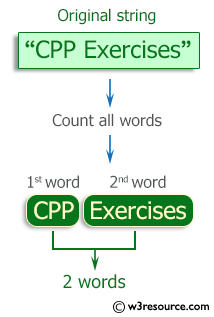
Sample Solution:
C++ Code :
#include <iostream> // Including input/output stream library
#include <string> // Including string handling library
using namespace std; // Using the standard namespace
// Function to count the number of words in a string
int Word_count(string text) {
int ctr = 0; // Initializing a counter variable to count words
// Loop through the string and count spaces to determine words
for (int x = 0; x < text.length(); x++) {
if (text[x] == ' ') // Checking for spaces to count words
ctr++; // Increment the counter for each space found
}
return ctr + 1; // Return the count of words by adding 1 to the total number of spaces (plus 1 for the last word without a trailing space)
}
// Main function
int main() {
// Displaying the count of words in different strings
cout << "Original string: Python, number of words -> " << Word_count("Python") << endl;
cout << "\nOriginal string: CPP Exercises, number of words -> " << Word_count("CPP Exercises") << endl;
cout << "\nOriginal string: After eagling the Road Hole on Thursday, he missed an 8-footer for birdie Friday., \nnumber of words -> ";
cout << Word_count("After eagling the Road Hole on Thursday, he missed an 8-footer for birdie Friday.") << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Original string: Python, number of words -> 1 Original string: CPP Exercises, number of words -> 2 Original string: After eagling the Road Hole on Thursday, he missed an 8-footer for birdie Friday., number of words -> 14
Flowchart:
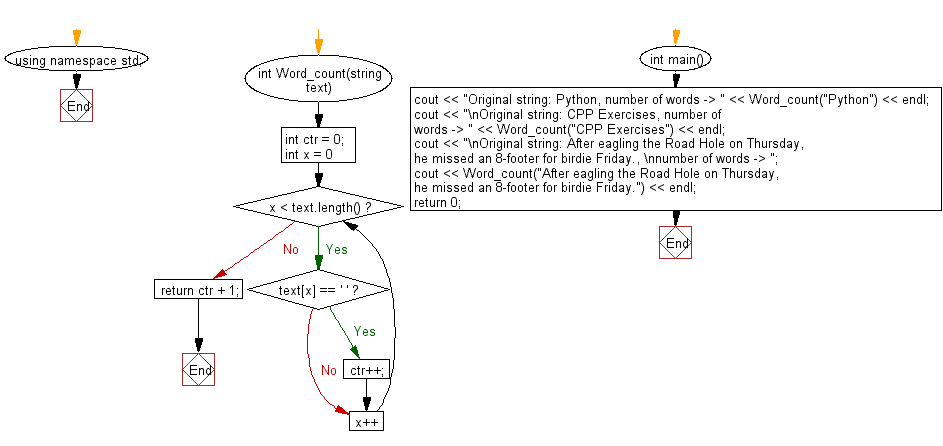
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Count all the vowels in a given string.
Next C++ Exercise: Check if two characters present equally in a string.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/string/cpp-string-exercise-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics