C++ String Exercises: Count all the vowels in a given string
7. Count Vowels in a String
Write a C++ program to count all the vowels in a given string.
Visual Presentation:
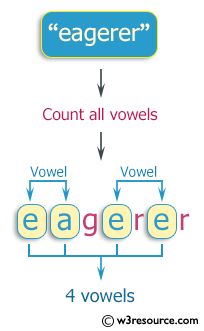
Sample Solution:
C++ Code :
#include <iostream> // Including input/output stream library
using namespace std; // Using the standard namespace
// Function to count the number of vowels in a string
int Vowel_Count(string text) {
int ctr = 0; // Initializing a counter variable to count vowels
// Loop through the string and count vowels (both lowercase and uppercase)
for (int i = 0; i < int(text.size()); i++) {
// Checking for lowercase vowels
if (text[i] == 'a' || text[i] == 'e' || text[i] == 'i' || text[i] == 'o' || text[i] == 'u')
++ctr; // Increment the counter if a lowercase vowel is found
// Checking for uppercase vowels
if (text[i] == 'A' || text[i] == 'E' || text[i] == 'I' || text[i] == 'O' || text[i] == 'U')
++ctr; // Increment the counter if an uppercase vowel is found
}
return ctr; // Return the total count of vowels in the string
}
// Main function
int main() {
// Checking the count of vowels in different strings and displaying the result
cout << "Original string: eagerer, number of vowels -> " << Vowel_Count("eagerer") << endl;
cout << "\nOriginal string: eaglets, number of vowels -> " << Vowel_Count("eaglets") << endl;
cout << "\nOriginal string: w3resource, number of vowels -> " << Vowel_Count("w3resource") << endl;
cout << "\nOriginal string: After eagling the Road Hole on Thursday, he missed an 8-footer for birdie Friday., number of vowels -> ";
cout << Vowel_Count("After eagling the Road Hole on Thursday, he missed an 8-footer for birdie Friday.") << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Original string: eagerer, number of vowels -> 4 Original string: eaglets, number of vowels -> 3 Original string: w3resource, number of vowels -> 4
Flowchart:
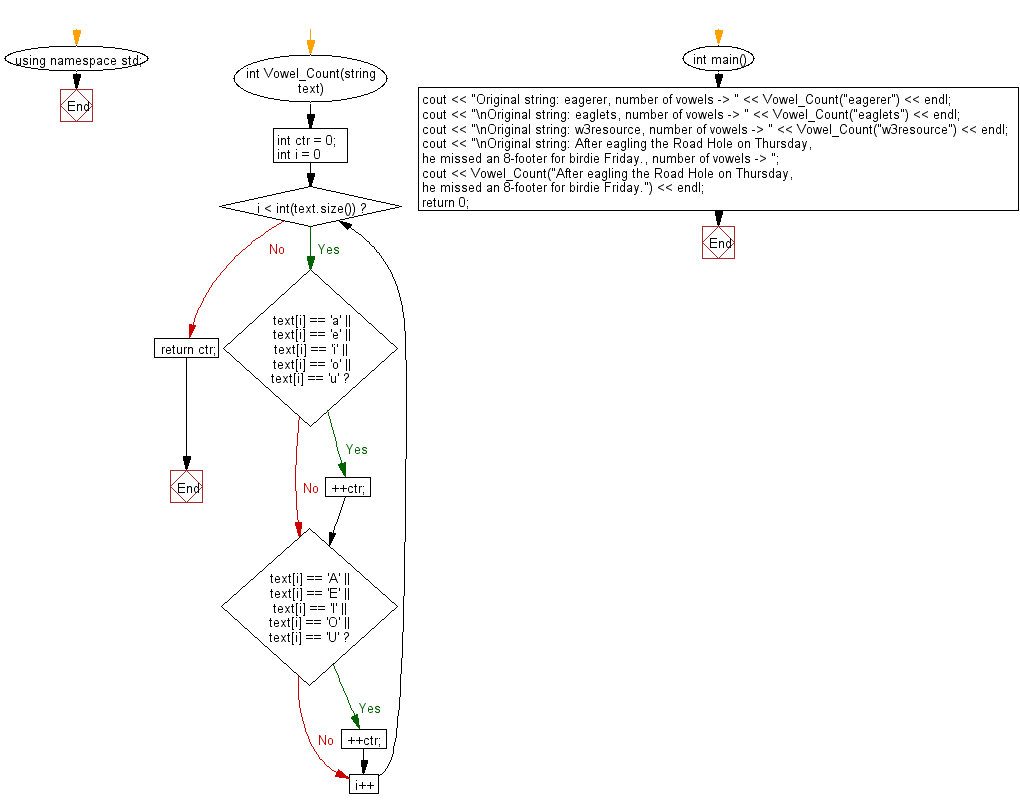
For more Practice: Solve these Related Problems:
- Write a C++ program to count all vowels in a string, considering both uppercase and lowercase.
- Write a C++ program that reads a string and uses a loop to tally vowels, outputting the final count.
- Write a C++ program to determine the total number of vowels in a string by iterating through each character and comparing ASCII values.
- Write a C++ program that converts a string to lowercase and then counts the vowels using a switch-case construct.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: If 2 characters are separated by 2 places in a string.
Next C++ Exercise: Count all the words in a given string.
What is the difficulty level of this exercise?