C++ String Exercises: If 2 characters are separated by 2 places in a string
Write a C++ program to check whether the characters e and g are separated by exactly 2 places anywhere in a given string at least once.
Sample Solution:
C++ Code :
#include <iostream> // Including input/output stream library
using namespace std; // Using the standard namespace
// Function to check for 'e', 'g', 'E', 'G' pattern in the string
bool Check_chars(string text) {
int len = int(text.size()); // Getting the length of the string
// Looping through the string to check for the pattern
for (int i = 0; i < len; i++) {
if (text[i] == 'e' || text[i] == 'E') { // Check for 'e' or 'E'
// Check if the characters at i+2 index form 'g' or 'G'
if (i + 2 < len && (text[i + 2] == 'g' || text[i + 2] == 'G'))
return true; // Return true if the pattern is found
}
if (text[i] == 'g' || text[i] == 'G') { // Check for 'g' or 'G'
// Check if the characters at i+2 index form 'e' or 'E'
if (i + 2 < len && (text[i + 2] == 'e' || text[i + 2] == 'E'))
return true; // Return true if the pattern is found
}
}
return false; // Return false if the pattern is not found in the entire string
}
// Main function
int main() {
// Checking the pattern in different strings and displaying the result
cout << "Original string: eagerer -> " << Check_chars("eagerer") << endl;
cout << "\nOriginal string: eaglets -> " << Check_chars("eaglets") << endl;
cout << "\nOriginal string: eardrop -> " << Check_chars("eardrop") << endl;
cout << "\nOriginal string: After eagling the Road Hole on Thursday, he missed an 8-footer for birdie Friday. -> ";
cout << Check_chars("After eagling the Road Hole on Thursday, he missed an 8-footer for birdie Friday.") << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Original string: eagerer -> 1 Original string: eaglets -> 1 Original string: eardrop -> 0
Flowchart:
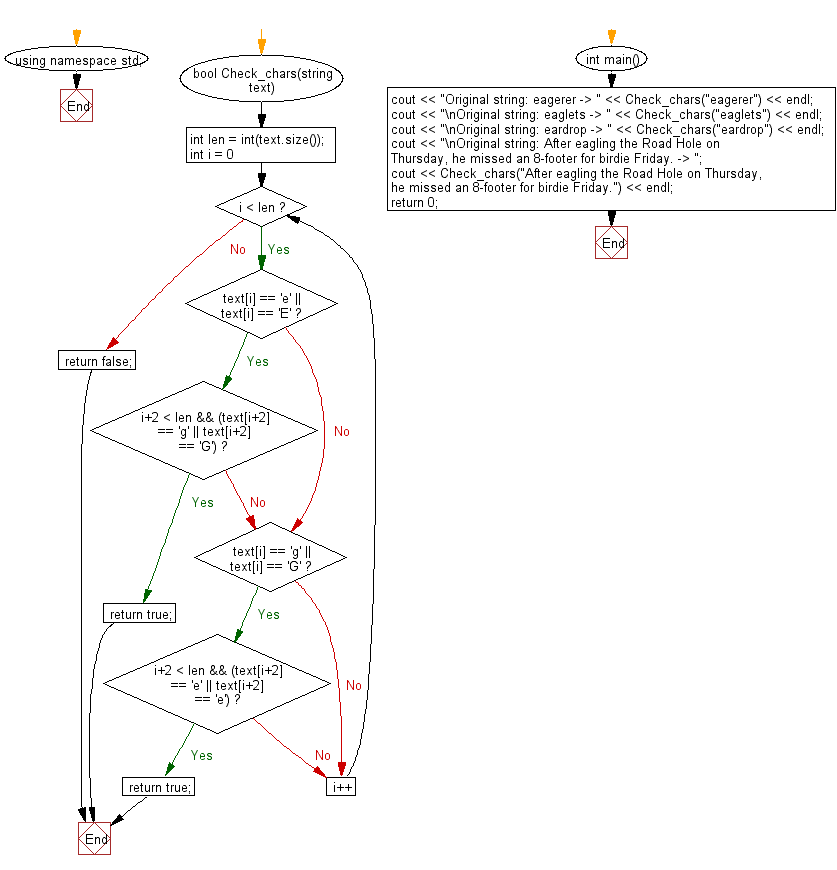
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Sort characters in a string.
Next C++ Exercise: Count all the vowels in a given string.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics