C++ String Exercises: Sort characters in a string
Write a C++ program to sort characters (numbers and punctuation symbols are not included) in a string.
Visual Presentation:
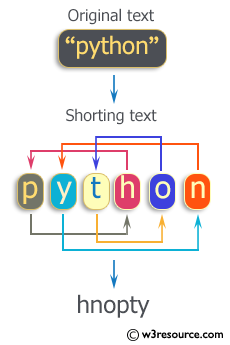
Sample Solution:
C++ Code :
#include <iostream> // Including input/output stream library
#include <string> // Including string library for string manipulation
using namespace std; // Using the standard namespace
// Function to sort characters in a string in ascending order and remove spaces
string sort_characters(string text) {
bool flag; // Flag for sorting loop
char ch; // Temporary character for swapping
// Loop to perform Bubble Sort
do
{
flag = false; // Resetting flag to false at the beginning of each pass
// Iterating through each character in the string
for (int x = 0; x < text.length() - 1; x++)
{
// Comparing adjacent characters and swapping if necessary to sort in ascending order
if (text[x] > text[x + 1])
{
ch = text[x];
text[x] = text[x + 1];
text[x + 1] = ch;
flag = true; // Set flag if a swap occurs
}
}
} while (flag); // Continue looping until no more swaps are needed (flag remains false)
// Remove spaces from the sorted string
string str; // Temporary string to store the sorted string without spaces
for (int y = 0; y < text.length(); y++)
{
if (text[y] != ' ')
{
str.push_back(text[y]); // Append non-space characters to the temporary string
}
}
return str; // Return the sorted string without spaces
}
int main() {
// Display original text and sorted text without spaces
cout << "Original text: python \nSorted text: ";
cout << sort_characters("python") << endl;
// Display another original text and its sorted version without spaces
cout << "\nOriginal text: AaBb \nSorted text: ";
cout << sort_characters("AaBb") << endl;
// Display another original text and its sorted version without spaces
cout << "\nOriginal text: the best way we learn anything is by practice and exercise questions \nSorted text: ";
cout << sort_characters("the best way we learn anything is by practice and exercise questions") << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Original text: python Sorted text: hnopty Original text: AaBb Sorted text: ABab Original text: the best way we learn anything is by practice and exercise questions Sorted text: aaaaabbcccdeeeeeeeeeghhiiiiilnnnnnopqrrrssssstttttuwwxyyy
Flowchart:
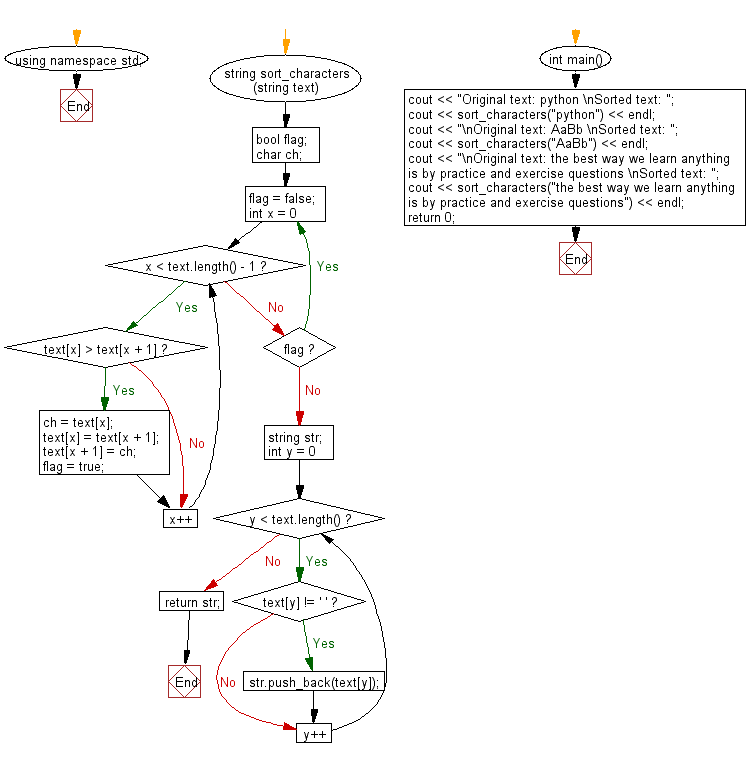
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Find the largest word in a given string.
Next C++ Exercise: If 2 characters are separated by 2 places in a string.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics