C++ String Exercises: Alternate the case of each letter in a string
C++ String: Exercise-42 with Solution
Write a C++ program that alternates the case of each letter in a given string of letters.
Pattern: First lowercase letter then uppercase letter and so on.
Test Data:
("JavaScript") -> "jAvAsCrIpT"
("Python") -> "pYtHoN"
("C++") -> "c++"
Sample Solution:
C++ Code:
#include <bits/stdc++.h> // Include all standard libraries
using namespace std; // Using the standard namespace
// Function to alternate the case of each letter in a given string
std::string test(std::string text) {
std::string str; // Initialize an empty string to store the modified text
int ctr = 0; // Initialize a counter variable
// Loop through each character in the input text
for (char ch : text) {
if (ctr % 2 == 0) // If the counter is even
str += tolower(ch); // Convert the character to lowercase and append to the result string
if (ctr % 2 != 0) // If the counter is odd
str += toupper(ch); // Convert the character to uppercase and append to the result string
if (ch == ' ') // If the character is a space
ctr++; // Increment the counter
ctr++; // Increment the counter after every character
}
return str; // Return the modified string
}
int main() {
// Test cases
string text = "JavaScript";
cout << "Original String: " << text;
cout << "\nAlternate the case of each letter in the said string: " << test(text);
text = "Python";
cout << "\n\nOriginal String: " << text;
cout << "\nAlternate the case of each letter in the said string: " << test(text);
text = "C++";
cout << "\n\nOriginal String: " << text;
cout << "\nAlternate the case of each letter in the said string: " << test(text);
}
Sample Output:
Original String: JavaScript Alternate the case of each letter in the said string: jAvAsCrIpT Original String: Python Alternate the case of each letter in the said string: pYtHoN Original String: C++ Alternate the case of each letter in the said string: c++
Flowchart:
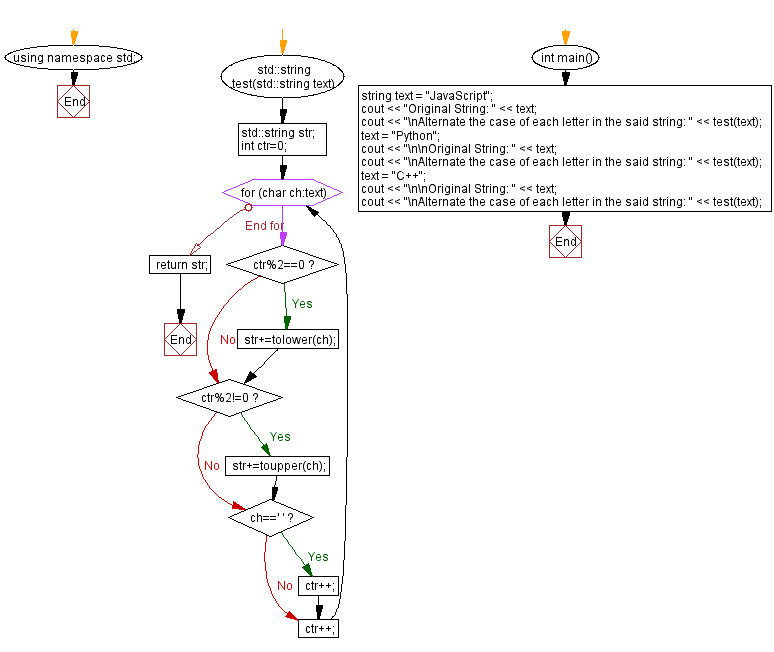
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Second occurrence of a string within another string.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics