C++ String Exercises: Second occurrence of a string within another string
C++ String: Exercise-41 with Solution
Write a C++ program that finds the position of the second occurrence of a string in another string. If the substring does not appear at least twice return -1.
Test Data:
("the qu qu", "qu") -> 7
("theququ", "qu") -> 5
("thequ", "qu") -> -1
Sample Solution:
C++ Code:
#include <bits/stdc++.h> // Include all standard libraries
using namespace std; // Using the standard namespace
// Function to find the position of the second occurrence of a substring in a string
int test(std::string text, std::string sstr) {
// Find the position of the first occurrence of the substring in the text
// Then, search for the substring from the index after the first occurrence
return text.find(sstr, text.find(sstr) + sstr.size());
}
int main() {
string text = "the qu qu"; // Initialize the input string
string sstr = "qu"; // Initialize the substring to search
cout << "String: " << text; // Display the original string
cout << "\nSubstring: " << sstr; // Display the substring
// Find and display the position of the second occurrence of the substring
cout << "\nPosition of the second occurrence of the said substring: " << test(text, sstr);
text = "theququ"; // Change the input string
sstr = "qu"; // Change the substring to search
cout << "\n\nString: " << text; // Display the modified string
cout << "\nSubstring: " << sstr; // Display the substring
// Find and display the position of the second occurrence of the substring
cout << "\nPosition of the second occurrence of the said substring: " << test(text, sstr);
text = "thequ"; // Change the input string
sstr = "qu"; // Change the substring to search
cout << "\n\nString: " << text; // Display the modified string
cout << "\nSubstring: " << sstr; // Display the substring
// Find and display the position of the second occurrence of the substring
cout << "\nPosition of the second occurrence of the said substring: " << test(text, sstr);
}
Sample Output:
String: the qu qu Substring: qu Position of the second occurrence of the said substring: 7 String: theququ Substring: qu Position of the second occurrence of the said substring: 5 String: thequ Substring: qu Position of the second occurrence of the said substring: -1
Flowchart:
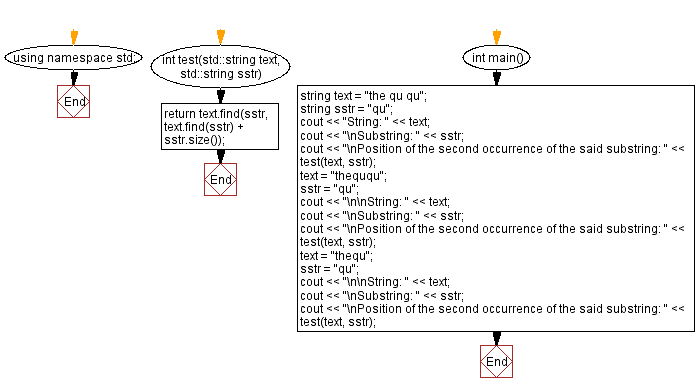
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Select lowercase characters from the other string.
Next C++ Exercise: Alternate the case of each letter in a string.What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/string/cpp-string-exercise-41.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics