C++ String Exercises: Select lowercase characters from the other string
40. Select Lowercase Characters at the Same Position from Two Strings
For two given strings, str1 and str2, write a C++ program to select only the characters that are lowercase in the other string at the same position. Return characters as a single string.
Test Data:
("Java", "jscript") -> "scr"
("jScript", "Java") -> "Jva"
("cpp", "c++") -> "c++"
Sample Solution:
C++ Code:
#include <bits/stdc++.h> // Include all standard libraries
using namespace std; // Using the standard namespace
// Function to concatenate characters at the same index if one string contains lowercase characters and the other doesn't
std::string test(std::string str1, std::string str2) {
int str_size;
// Determine the smaller size between the two input strings
str1.size() < str2.size() ? str_size = str1.size() : str_size = str2.size();
std::string s1 = ""; // Initialize an empty string s1
std::string s2 = ""; // Initialize an empty string s2
std::string result = ""; // Initialize an empty string result to store the final concatenated string
// Iterate through the strings up to the determined size
for (int i = 0; i < str_size; i++) {
if (str1[i] >= 'a' && str1[i] <= 'z') // Check if str1 has a lowercase character
s2.push_back(str2[i]); // Add the character from str2 to s2 at the same index
if (str2[i] >= 'z' && str2[i] <= 'z') // Check if str2 has a lowercase character
s1.push_back(str1[i]); // Add the character from str1 to s1 at the same index
}
result = s1 + s2; // Concatenate s1 and s2 to form the final result
return result; // Return the concatenated string
}
int main() {
// Test cases
string str1 = "Java";
string str2 = "jscript";
cout << "Strings: " << str1 << " " << str2;
cout << "\nCheck said string contains lowercase characters! " << test(str1, str2) << endl;
str1 = "jScript";
str2 = "Java";
cout << "Strings: " << str1 << " " << str2;
cout << "\nCheck said string contains lowercase characters! " << test(str1, str2) << endl;
str1 = "cpp";
str2 = "c++";
cout << "Strings: " << str1 << " " << str2;
cout << "\nCheck said string contains lowercase characters! " << test(str1, str2) << endl;
}
Sample Output:
Strings: Java jscript Check said string contains lowercase characters! scr Strings: jScript Java Check said string contains lowercase characters! Jva Strings: cpp c++ Check said string contains lowercase characters! c++
Flowchart:
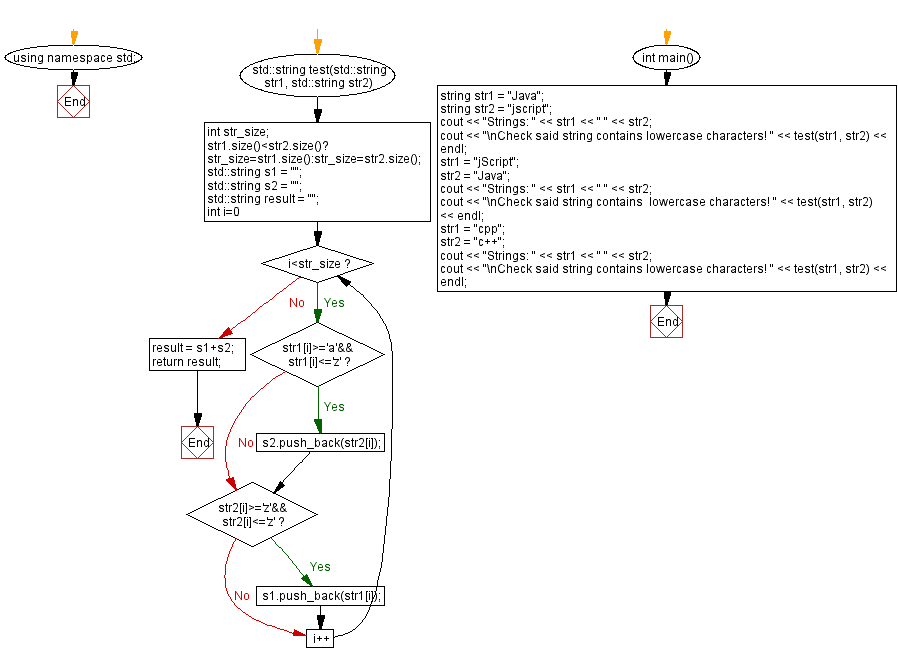
For more Practice: Solve these Related Problems:
- Write a C++ program to compare two strings and construct a new string using only the lowercase characters from the second string at positions where the first string has uppercase letters.
- Write a C++ program that reads two strings and returns a string formed by selecting characters from one string where the corresponding character in the other string is lowercase.
- Write a C++ program to process two input strings and output a new string by extracting lowercase letters from the longer string at positions where the other string has letters.
- Write a C++ program to alternate between two strings by selecting characters that are lowercase in the other string at the same index, then concatenating the result.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Find Unique Character String.
Next C++ Exercise: Second occurrence of a string within another string.What is the difficulty level of this exercise?