C++ String Exercises: Find the largest word in a given string
4. Find the Largest Word in a String
Write a C++ program to find the largest word in a given string.
Visual Presentation:
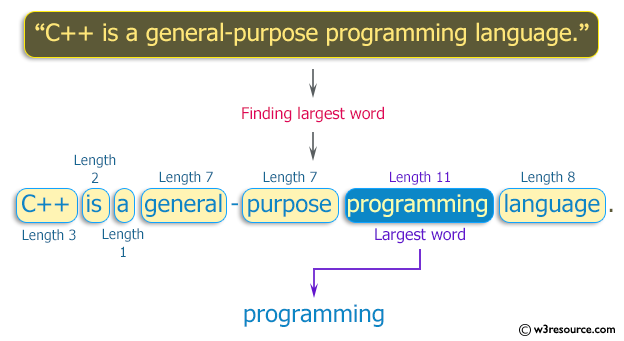
Sample Solution:
C++ Code :
Sample Output:
Original String: C++ is a general-purpose programming language. Longest word: programming Original String: The best way we learn anything is by practice and exercise questions. Longest word: questions Original String: Hello Longest word: Hello
Flowchart:
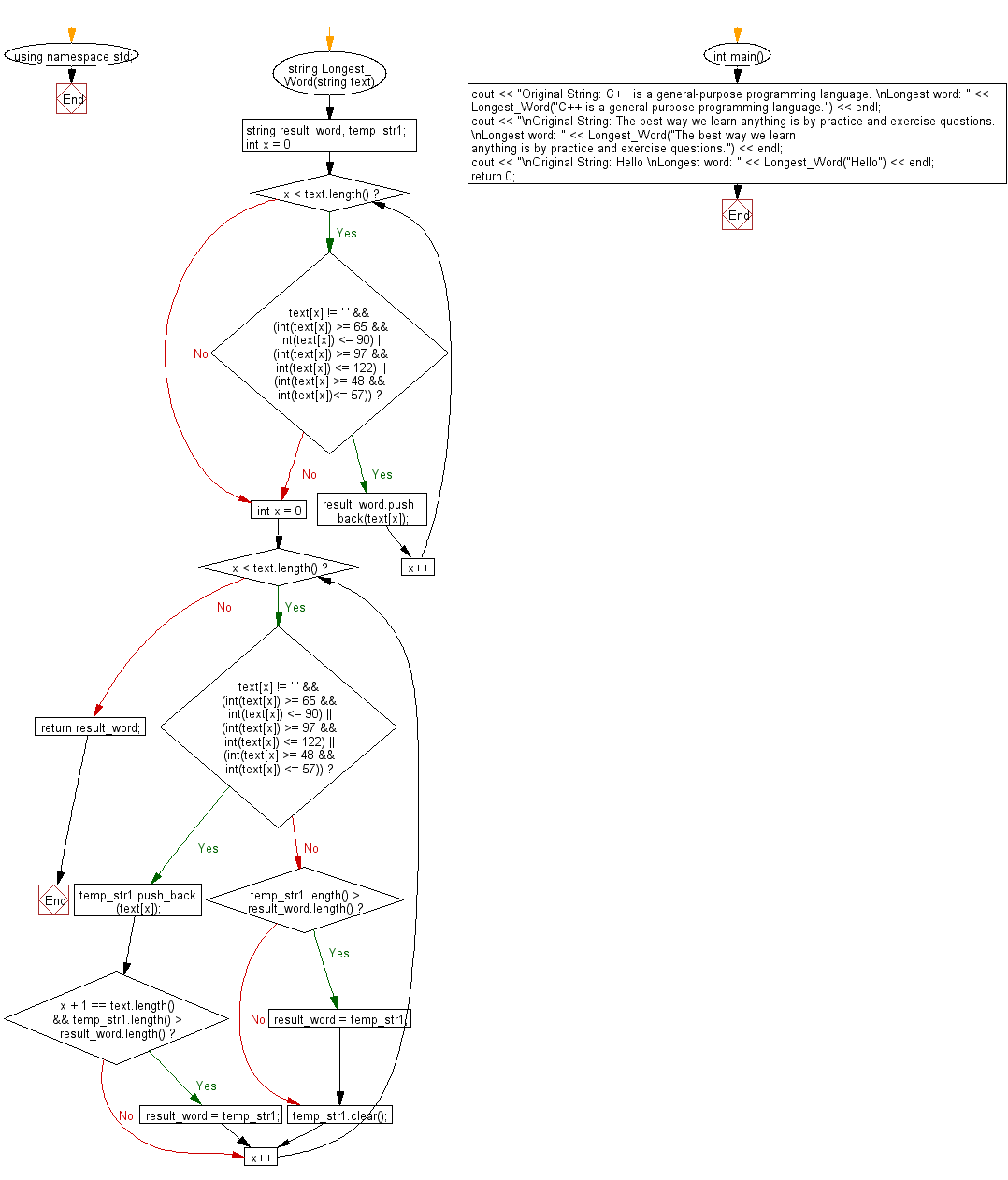
For more Practice: Solve these Related Problems:
- Write a C++ program to identify and print the longest word in a sentence, ignoring punctuation.
- Write a C++ program that finds the longest word in a string by comparing lengths after splitting by whitespace.
- Write a C++ program to output the longest word from a given string, and if there are multiple, print the first occurrence.
- Write a C++ program to scan a sentence and return the word with the maximum length, excluding non-letter characters.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Capitalize the first letter of each word of a string.
Next C++ Exercise: Sort characters in a string.
What is the difficulty level of this exercise?