C++ String Exercises: Find Unique Character String
39. Check if a String Contains Only Unique Characters
Write a C++ program that checks whether a given string contains unique characters or not. Return true if the string contains unique characters otherwise false.
Test Data:
("Filename") -> 0
("abc") -> 1
Sample Solution:
C++ Code:
#include <bits/stdc++.h> // Include all standard libraries
using namespace std; // Using the standard namespace
// Function to check if a string contains all unique characters
bool test(string word) {
for (int i = 0; i < word.length() - 1; i++) { // Iterate through the characters in the string
for (int j = i + 1; j < word.length(); j++) { // Check against subsequent characters in the string
if (word[i] == word[j]) { // If a character repeats later in the string
return false; // Return false, indicating the string doesn't contain all unique characters
}
}
}
return true; // Return true if no characters are repeated, indicating all characters are unique
}
int main() {
// Test cases
string str = "Filename"; // Define a string
cout << "String: " << str;
cout << "\nCheck said string contains unique characters! " << test(str) << endl; // Display if "Filename" contains all unique characters
str = "abc"; // Define another string
cout << "\nString: " << str;
cout << "\nCheck said string contains unique characters! " << test(str) << endl; // Display if "abc" contains all unique characters
}
Sample Output:
String: Filename Check said string contains unique characters! 0 String: abc Check said string contains unique characters! 1
Flowchart:
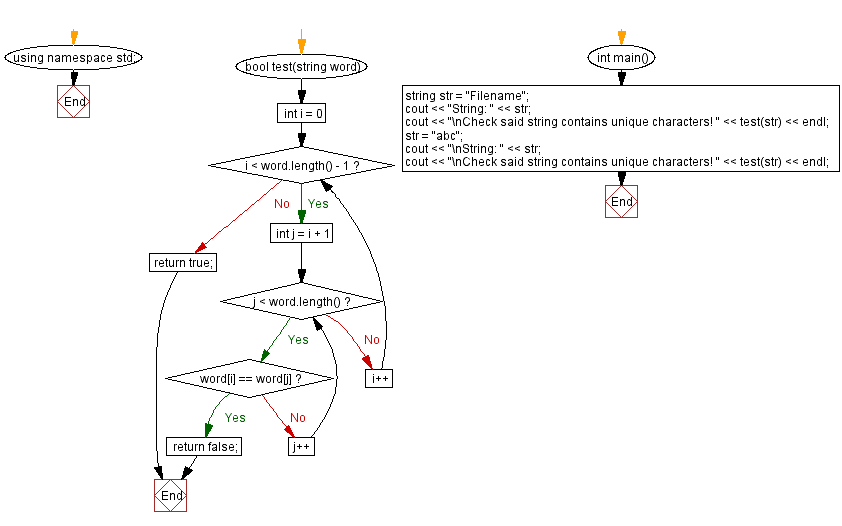
For more Practice: Solve these Related Problems:
- Write a C++ program to determine if a string has all unique characters by comparing each character with every other character.
- Write a C++ program that uses a boolean array to check if all characters in a string are distinct.
- Write a C++ program to check uniqueness of characters in a string without using any STL containers.
- Write a C++ program that reads a string and returns true if no character appears more than once, otherwise false.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Remove a specific character from a given string.
Next C++ Exercise: Select lowercase characters from the other string.What is the difficulty level of this exercise?