C++ String Exercises: Count occurrences of a certain character in a string
Write a C++ program that counts the number of instances of a certain character in a given string.
Test Data:
("Exercises", "e") -> 2
("Compilation Time", "i") -> 3
Sample Solution:
C++ Code:
#include <bits/stdc++.h> // Include all standard libraries
using namespace std; // Using the standard namespace
// Function to count occurrences of a specific character in a given string
int test(string s, char c) {
int res = 0; // Initialize a variable to count occurrences of the character
for (int i = 0; i < s.length(); i++) { // Loop through each character of the string
if (s[i] == c) { // Check if the current character matches the specified character
res++; // Increment the count of occurrences if a match is found
}
}
return res; // Return the total count of occurrences
}
int main() {
// Test cases
string str = "Exercises"; // Define a string
char ch = 'e'; // Define a character
cout << "String: " << str;
cout << "\nCharacter: " << ch;
cout << "\nCount occurrences of '" << ch << "' in '" << str << "': " << test(str, ch) << endl; // Display the count of 'e' in "Exercises"
str = "Compilation Time"; // Update the string
ch = 'i'; // Update the character
cout << "\nString: " << str;
cout << "\nCharacter: " << ch;
cout << "\nCount occurrences of '" << ch << "' in '" << str << "': " << test(str, ch) << endl; // Display the count of 'i' in "Compilation Time"
}
Sample Output:
String: Exercises Character: e Count occurrences of 'e' in 'Exercises': 2 String: Compilation Time Character: i Count occurrences of 'i' in 'Compilation Time': 3
Flowchart:
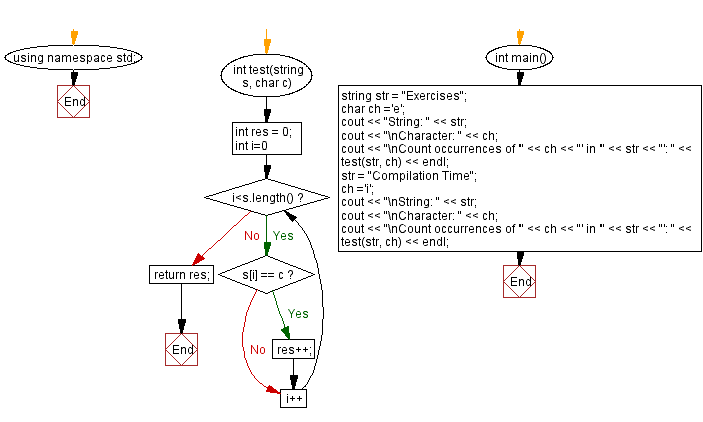
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Check two consecutive, identical letters in a word.
Next C++ Exercise: Remove a specific character from a given string.What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics